【问题描述】读入一个C程序,统计程序中代码、注释和空行的行数以及函数的个数和平均行数,并利用统计信息分析评价该程序的风格。【基本要求】(1) 把 C 程序文件按字符顺序读入源程序;(2) 边读入程序,边识别统计代码行、注释行和空行,同时还要识别函数的开始和结束,以便统计其个数和平均行数。(3) 程序的风格评价分为代码、注释和空行三个方面。每个方面分为 A,B,C 和 D 四个等级 , 等级的划分标准是 : A级 B级 C级 D级 代码(函数平均长度) 10~15行 8~9或16~20行 5~7或21~24行 <5或>24行 注释(占总行数比率) 15~25% 10~14或26~30% 5~9或31~35% <5%或>35% 空行(占总行数比率) 15~25% 10~14或26~30% 5~9或31~35% <5%或>35% 用c++编写
时间: 2023-06-17 11:05:58 浏览: 391
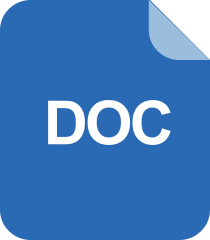
读入一个C程序,统计程序中代码、注释和空行的行数以及函数的个数和平行行数
以下是一个可能的实现,代码中有注释解释每个步骤的具体操作。
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <regex>
using namespace std;
int main() {
// 打开C程序文件
ifstream fin("program.c");
if (!fin.is_open()) {
cout << "Failed to open file!" << endl;
return 0;
}
// 读入文件中的每一行
string line;
vector<string> lines; // 存储每一行
while (getline(fin, line)) {
lines.push_back(line);
}
// 统计各种行数和函数信息
int codeLines = 0; // 代码行数
int commentLines = 0; // 注释行数
int blankLines = 0; // 空行数
int functionCount = 0; // 函数个数
int totalFunctionLines = 0; // 所有函数的总行数
bool isInFunction = false; // 是否正在处理函数
int currentFunctionLines = 0; // 当前处理的函数的行数
for (int i = 0; i < lines.size(); i++) {
string currentLine = lines[i];
// 判断当前行是否为空行
if (regex_match(currentLine, regex("^\\s*$"))) {
blankLines++;
continue;
}
// 判断当前行是否为注释行
if (regex_match(currentLine, regex("^\\s*//.*$"))) {
commentLines++;
continue;
}
if (regex_match(currentLine, regex("^\\s*/\\*.*$"))) {
commentLines++;
// 如果当前行是多行注释的第一行,则一直往下读取,直到找到多行注释的结束
while (!regex_match(currentLine, regex(".*\\*/\\s*$"))) {
i++;
currentLine = lines[i];
commentLines++;
}
continue;
}
// 如果当前行不是空行或注释行,则认为是代码行
codeLines++;
// 判断是否进入函数定义
if (!isInFunction && regex_match(currentLine, regex("^\\s*\\w+\\s+\\w+\\(.*\\)\\s*\\{.*$"))) {
isInFunction = true;
functionCount++;
currentFunctionLines = 1;
continue;
}
// 如果正在处理函数,则判断是否到达函数结束
if (isInFunction) {
currentFunctionLines++;
if (regex_match(currentLine, regex("^\\s*\\}.*$"))) {
isInFunction = false;
totalFunctionLines += currentFunctionLines;
}
}
}
// 计算统计信息
int totalLines = lines.size();
double codeRatio = (double) codeLines / totalLines;
double commentRatio = (double) commentLines / totalLines;
double blankRatio = (double) blankLines / totalLines;
double averageFunctionLines = (double) totalFunctionLines / functionCount;
// 输出统计信息
cout << "Total lines: " << totalLines << endl;
cout << "Code lines: " << codeLines << endl;
cout << "Comment lines: " << commentLines << endl;
cout << "Blank lines: " << blankLines << endl;
cout << "Function count: " << functionCount << endl;
cout << "Average function lines: " << averageFunctionLines << endl;
// 输出风格评价
char codeStyle, commentStyle, blankStyle;
if (averageFunctionLines >= 10 && averageFunctionLines <= 15) {
codeStyle = 'A';
} else if ((averageFunctionLines >= 8 && averageFunctionLines <= 9) || (averageFunctionLines >= 16 && averageFunctionLines <= 20)) {
codeStyle = 'B';
} else if ((averageFunctionLines >= 5 && averageFunctionLines <= 7) || (averageFunctionLines >= 21 && averageFunctionLines <= 24)) {
codeStyle = 'C';
} else {
codeStyle = 'D';
}
if (commentRatio >= 0.15 && commentRatio <= 0.25) {
commentStyle = 'A';
} else if ((commentRatio >= 0.1 && commentRatio <= 0.14) || (commentRatio >= 0.26 && commentRatio <= 0.3)) {
commentStyle = 'B';
} else if ((commentRatio >= 0.05 && commentRatio <= 0.09) || (commentRatio >= 0.31 && commentRatio <= 0.35)) {
commentStyle = 'C';
} else {
commentStyle = 'D';
}
if (blankRatio >= 0.15 && blankRatio <= 0.25) {
blankStyle = 'A';
} else if ((blankRatio >= 0.1 && blankRatio <= 0.14) || (blankRatio >= 0.26 && blankRatio <= 0.3)) {
blankStyle = 'B';
} else if ((blankRatio >= 0.05 && blankRatio <= 0.09) || (blankRatio >= 0.31 && blankRatio <= 0.35)) {
blankStyle = 'C';
} else {
blankStyle = 'D';
}
cout << "Code style: " << codeStyle << endl;
cout << "Comment style: " << commentStyle << endl;
cout << "Blank style: " << blankStyle << endl;
return 0;
}
```
阅读全文
相关推荐
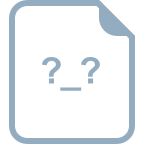


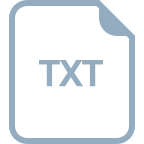
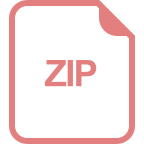
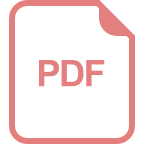
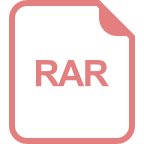
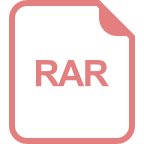
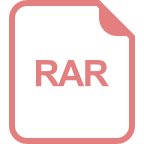
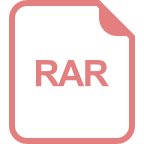
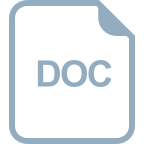
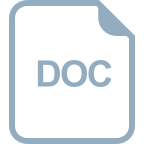
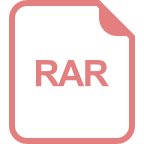
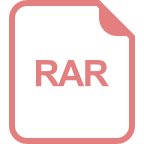
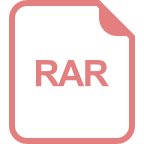
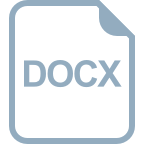