用SSM实现图书管理系统的图书类型管理模块用代码写出
时间: 2023-12-14 21:37:44 浏览: 29
以下是使用SSM框架实现图书管理系统的图书类型管理模块的代码示例:
1. 对应的Mapper接口:BookTypeMapper.java
```java
package com.example.demo.mapper;
import com.example.demo.pojo.BookType;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Param;
import java.util.List;
@Mapper
public interface BookTypeMapper {
int insert(BookType record);
int insertSelective(BookType record);
int updateByPrimaryKeySelective(BookType record);
int updateByPrimaryKey(BookType record);
int deleteByPrimaryKey(Integer id);
List<BookType> selectAllBookTypes();
BookType selectByPrimaryKey(Integer id);
List<BookType> selectBookTypesByKeyword(@Param("keyword") String keyword);
}
```
2. 对应的Service接口:BookTypeService.java
```java
package com.example.demo.service;
import com.example.demo.pojo.BookType;
import java.util.List;
public interface BookTypeService {
int addBookType(BookType bookType);
int updateBookType(BookType bookType);
int deleteBookType(Integer id);
List<BookType> getAllBookTypes();
BookType getBookTypeById(Integer id);
List<BookType> getBookTypesByKeyword(String keyword);
}
```
3. 对应的Service实现类:BookTypeServiceImpl.java
```java
package com.example.demo.service.impl;
import com.example.demo.mapper.BookTypeMapper;
import com.example.demo.pojo.BookType;
import com.example.demo.service.BookTypeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookTypeServiceImpl implements BookTypeService {
@Autowired
private BookTypeMapper bookTypeMapper;
@Override
public int addBookType(BookType bookType) {
return bookTypeMapper.insertSelective(bookType);
}
@Override
public int updateBookType(BookType bookType) {
return bookTypeMapper.updateByPrimaryKeySelective(bookType);
}
@Override
public int deleteBookType(Integer id) {
return bookTypeMapper.deleteByPrimaryKey(id);
}
@Override
public List<BookType> getAllBookTypes() {
return bookTypeMapper.selectAllBookTypes();
}
@Override
public BookType getBookTypeById(Integer id) {
return bookTypeMapper.selectByPrimaryKey(id);
}
@Override
public List<BookType> getBookTypesByKeyword(String keyword) {
return bookTypeMapper.selectBookTypesByKeyword(keyword);
}
}
```
4. 对应的Controller类:BookTypeController.java
```java
package com.example.demo.controller;
import com.example.demo.pojo.BookType;
import com.example.demo.service.BookTypeService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@Controller
@RequestMapping("/bookType")
public class BookTypeController {
@Autowired
private BookTypeService bookTypeService;
@GetMapping("/list")
public String list(Model model) {
List<BookType> bookTypes = bookTypeService.getAllBookTypes();
model.addAttribute("bookTypes", bookTypes);
return "bookType/list";
}
@GetMapping("/add")
public String add(Model model) {
model.addAttribute("bookType", new BookType());
return "bookType/add";
}
@PostMapping("/add")
public String add(BookType bookType) {
bookTypeService.addBookType(bookType);
return "redirect:/bookType/list";
}
@GetMapping("/update/{id}")
public String update(@PathVariable("id") Integer id, Model model) {
BookType bookType = bookTypeService.getBookTypeById(id);
model.addAttribute("bookType", bookType);
return "bookType/update";
}
@PostMapping("/update")
public String update(BookType bookType) {
bookTypeService.updateBookType(bookType);
return "redirect:/bookType/list";
}
@GetMapping("/delete/{id}")
public String delete(@PathVariable("id") Integer id) {
bookTypeService.deleteBookType(id);
return "redirect:/bookType/list";
}
@GetMapping("/search")
public String search(@RequestParam(value = "keyword", required = false) String keyword, Model model) {
List<BookType> bookTypes;
if (keyword == null || keyword.equals("")) {
bookTypes = bookTypeService.getAllBookTypes();
} else {
bookTypes = bookTypeService.getBookTypesByKeyword(keyword);
}
model.addAttribute("bookTypes", bookTypes);
return "bookType/list";
}
}
```
5. 对应的页面文件:list.html、add.html、update.html
list.html:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>图书类型管理</title>
</head>
<body>
<h1>图书类型管理</h1>
<a href="/bookType/add">添加图书类型</a>
<form action="/bookType/search" method="get">
<input type="text" name="keyword" placeholder="请输入关键字">
<button type="submit">搜索</button>
</form>
<table border="1">
<tr>
<th>编号</th>
<th>名称</th>
<th>描述</th>
<th>操作</th>
</tr>
<tr th:each="bookType : ${bookTypes}">
<td th:text="${bookType.id}"></td>
<td th:text="${bookType.name}"></td>
<td th:text="${bookType.description}"></td>
<td>
<a th:href="@{/bookType/update/{id}(id=${bookType.id})}">修改</a>
<a th:href="@{/bookType/delete/{id}(id=${bookType.id})}" onclick="return confirm('确认删除?')">删除</a>
</td>
</tr>
</table>
</body>
</html>
```
add.html:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>添加图书类型</title>
</head>
<body>
<h1>添加图书类型</h1>
<form action="/bookType/add" method="post">
<label for="name">名称:</label>
<input type="text" id="name" name="name" required>
<br>
<label for="description">描述:</label>
<textarea id="description" name="description"></textarea>
<br>
<button type="submit">提交</button>
</form>
<a href="/bookType/list">返回列表</a>
</body>
</html>
```
update.html:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>修改图书类型</title>
</head>
<body>
<h1>修改图书类型</h1>
<form action="/bookType/update" method="post">
<input type="hidden" name="id" th:value="${bookType.id}">
<label for="name">名称:</label>
<input type="text" id="name" name="name" th:value="${bookType.name}" required>
<br>
<label for="description">描述:</label>
<textarea id="description" name="description" th:text="${bookType.description}"></textarea>
<br>
<button type="submit">提交</button>
</form>
<a href="/bookType/list">返回列表</a>
</body>
</html>
```
相关推荐
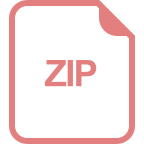
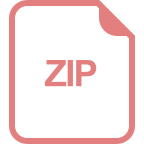
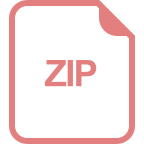











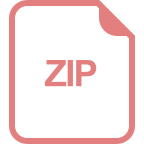
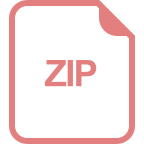
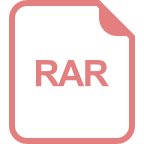