采用json格式文件存储unity对象数据
时间: 2023-08-24 14:59:29 浏览: 106
在Unity中,您可以使用JsonUtility类将Unity对象数据以JSON格式保存到文件中。JsonUtility类提供了一组静态方法,用于将对象序列化为JSON字符串,以及将JSON字符串反序列化为对象。
以下是一个示例代码,展示如何使用JsonUtility类将Unity对象数据保存到JSON文件中:
```csharp
using UnityEngine;
using System.IO;
[System.Serializable]
public class PlayerData
{
public string playerName;
public int playerLevel;
public float playerHealth;
}
public class JsonExample : MonoBehaviour
{
void Start()
{
// 创建一个PlayerData对象并设置数据
PlayerData playerData = new PlayerData();
playerData.playerName = "John";
playerData.playerLevel = 5;
playerData.playerHealth = 100f;
// 将PlayerData对象转换为JSON字符串
string json = JsonUtility.ToJson(playerData);
// 将JSON字符串写入文件
string filePath = Application.persistentDataPath + "/playerData.json";
File.WriteAllText(filePath, json);
Debug.Log("Player data saved to: " + filePath);
}
}
```
在上述示例代码中,我们先创建一个名为PlayerData的类,它包含了我们想要保存的对象数据。然后,在Start()方法中,我们创建一个PlayerData对象并设置数据。接下来,我们使用JsonUtility.ToJson()方法将PlayerData对象转换为JSON字符串。最后,我们使用File.WriteAllText()方法将JSON字符串写入文件。
请注意,我们使用了Application.persistentDataPath来获取一个可持久化的文件路径,以便在不同平台上都能正确保存文件。
要读取JSON文件并将其反序列化为对象,您可以使用JsonUtility.FromJson()方法。以下是一个示例代码:
```csharp
void LoadPlayerData()
{
string filePath = Application.persistentDataPath + "/playerData.json";
string json = File.ReadAllText(filePath);
PlayerData playerData = JsonUtility.FromJson<PlayerData>(json);
Debug.Log("Loaded player data: " + playerData.playerName + ", Level: " + playerData.playerLevel + ", Health: " + playerData.playerHealth);
}
```
在上述示例代码中,我们首先读取JSON文件中的内容,然后使用JsonUtility.FromJson()方法将其反序列化为PlayerData对象。
希望这个示例对您有所帮助!如有任何问题,请随时向我提问。
阅读全文
相关推荐
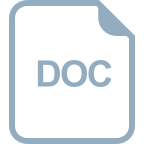
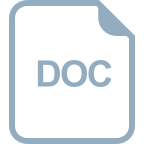
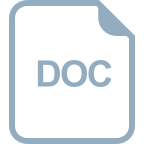
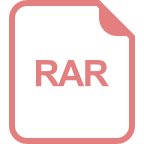
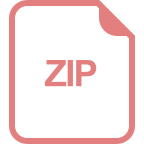
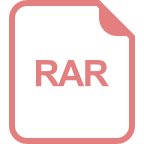
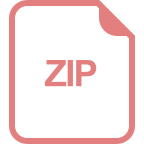











