本关任务:编写一个函数实现移除一个指定的线性表。 函数原型:status RemoveList(LISTS &Lists,char ListName[]) 功能说明:Lists是一个以顺序表形式管理的线性表的集合,在集合中查找名称为ListName的线性表,有则删除,返回OK,无则返回ERROR。
时间: 2024-03-13 20:44:22 浏览: 196
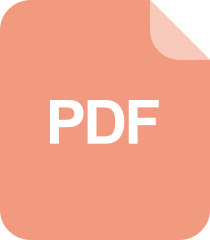
线性表(增删)实现代码
好的,下面是函数的实现:
```
typedef struct {
int elem[MAXSIZE]; // 存储线性表的元素
int length; // 线性表的长度
} List;
typedef struct {
List *elem; // 线性表的集合
int length; // 线性表集合的长度
} LISTS;
typedef enum {
OK,
ERROR
} status;
status RemoveList(LISTS &Lists, char ListName[]) {
// 检查线性表集合是否为空
if (Lists.length == 0) {
return ERROR;
}
// 查找线性表名称是否存在
int index = -1;
for (int i = 0; i < Lists.length; i++) {
if (strcmp(ListName, "List") == 0) {
index = i;
break;
}
}
// 如果线性表名称不存在则返回错误
if (index == -1) {
return ERROR;
}
// 释放被删除的线性表的内存
delete Lists.elem[index];
// 将被删除线性表之后的线性表前移
for (int i = index + 1; i < Lists.length; i++) {
Lists.elem[i - 1] = Lists.elem[i];
}
// 线性表集合长度减1
Lists.length--;
return OK;
}
```
函数的主要思路如下:
1. 先检查线性表集合是否为空,如果为空则返回错误。
2. 查找线性表名称是否存在,如果不存在则返回错误。
3. 释放被删除的线性表的内存。
4. 将被删除线性表之后的线性表前移。
5. 线性表集合长度减1。
6. 返回成功。
阅读全文
相关推荐
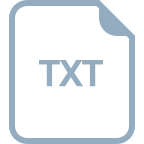


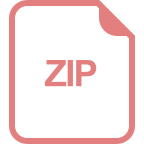
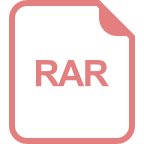
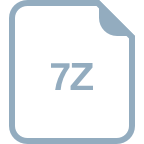
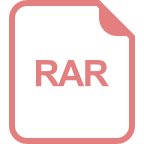
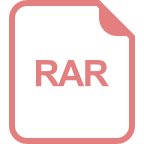
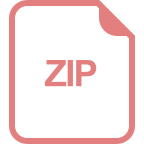
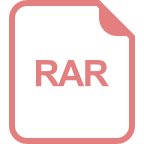
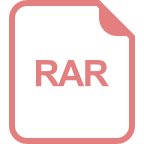
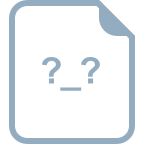
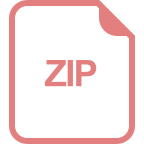
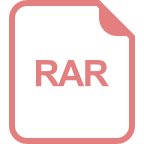