用 python 实现
时间: 2023-07-12 20:50:18 浏览: 64
好的,我先介绍一下 Python 中常用的 AI 库,然后再给你一个简单的示例。
Python 中常用的 AI 库有 TensorFlow、PyTorch、Keras 等,它们都提供了各种各样的 AI 模型和算法。其中,TensorFlow 是一个流行的深度学习库,它有一个高级的 API,可以使开发者更加容易地构建深度学习模型。PyTorch 是另一个流行的深度学习库,它的设计理念是简单、灵活和可扩展的。Keras 则是一个高度模块化、易于扩展的深度学习库,它可以运行在 TensorFlow、Theano 和 CNTK 等后端上。
现在,我给你一个简单的使用 TensorFlow 库实现的 AI 对话程序的示例:
```python
import tensorflow as tf
import numpy as np
# 定义模型参数
vocab_size = 10000
embedding_dim = 256
hidden_dim = 1024
num_layers = 4
# 构建模型
model = tf.keras.Sequential([
tf.keras.layers.Embedding(vocab_size, embedding_dim),
tf.keras.layers.LSTM(hidden_dim, return_sequences=True),
tf.keras.layers.LSTM(hidden_dim),
tf.keras.layers.Dense(vocab_size, activation='softmax')
])
# 编译模型
model.compile(optimizer='adam', loss='categorical_crossentropy')
# 加载模型权重
model.load_weights('model_weights.h5')
# 定义预处理函数
def preprocess(text):
# 将文本转换为数字序列
tokens = text.split()
token_ids = [word_to_id.get(token, 0) for token in tokens]
return np.array(token_ids)
# 定义生成回复的函数
def generate_reply(text):
# 预处理输入文本
input_seq = preprocess(text)
# 将输入文本转换为模型可接受的格式
input_seq = tf.expand_dims(input_seq, axis=0)
# 生成回复
output_seq = model.predict(input_seq)
output_seq = tf.squeeze(output_seq, axis=0)
output_seq = tf.argmax(output_seq, axis=-1)
output_seq = output_seq.numpy().tolist()
# 将数字序列转换为文本
reply = ' '.join([id_to_word.get(id, '') for id in output_seq])
return reply
# 测试
while True:
text = input('> ')
reply = generate_reply(text)
print(reply)
```
这个程序使用了一个基于 LSTM 的语言模型,可以根据输入的文本生成回复。其中,`preprocess` 函数将输入文本转换为数字序列,`generate_reply` 函数将数字序列转换为文本。程序的输入是用户输入的文本,输出是 AI 生成的回复。
阅读全文
相关推荐
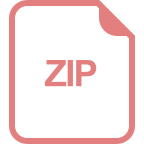
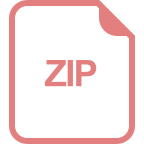
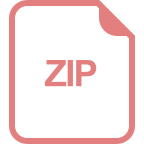















