C++中使用http的例子
时间: 2024-06-09 18:05:59 浏览: 8
以下是一个使用C++的libcurl库实现HTTP GET请求的例子:
```cpp
#include <iostream>
#include <curl/curl.h>
int main() {
CURL* curl;
CURLcode res;
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://www.example.com"); // 设置要请求的URL
res = curl_easy_perform(curl); // 执行请求
if (res != CURLE_OK) { // 检查请求是否成功
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl); // 释放资源
}
return 0;
}
```
如果需要发送POST请求,可以使用以下代码:
```cpp
#include <iostream>
#include <curl/curl.h>
int main() {
CURL* curl;
CURLcode res;
curl = curl_easy_init();
if (curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://www.example.com"); // 设置要请求的URL
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "data=some_data"); // 设置POST数据
res = curl_easy_perform(curl); // 执行请求
if (res != CURLE_OK) { // 检查请求是否成功
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
curl_easy_cleanup(curl); // 释放资源
}
return 0;
}
```
注意,以上代码需要安装libcurl库才能编译运行。
相关推荐
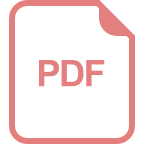















