C# 语言 快速排序
时间: 2023-06-28 18:12:19 浏览: 87
快速排序(Quick Sort)是一种基于比较的排序算法,它的时间复杂度为O(nlogn),常用于对数组进行排序。
以下是 C# 语言实现快速排序的示例代码:
```csharp
using System;
class QuickSort {
static void Main(string[] args) {
int[] arr = { 5, 2, 8, 6, 3, 7, 1, 4 };
Console.WriteLine("Original Array:");
PrintArray(arr);
QuickSortAlgorithm(arr, 0, arr.Length - 1);
Console.WriteLine("\nSorted Array:");
PrintArray(arr);
}
static void QuickSortAlgorithm(int[] arr, int left, int right) {
if (left < right) {
int pivotIndex = Partition(arr, left, right);
QuickSortAlgorithm(arr, left, pivotIndex - 1);
QuickSortAlgorithm(arr, pivotIndex + 1, right);
}
}
static int Partition(int[] arr, int left, int right) {
int pivot = arr[right];
int i = left - 1;
for (int j = left; j <= right - 1; j++) {
if (arr[j] < pivot) {
i++;
Swap(ref arr[i], ref arr[j]);
}
}
Swap(ref arr[i + 1], ref arr[right]);
return i + 1;
}
static void Swap(ref int a, ref int b) {
int temp = a;
a = b;
b = temp;
}
static void PrintArray(int[] arr) {
foreach (int element in arr) {
Console.Write(element + " ");
}
}
}
```
程序输出:
```
Original Array:
5 2 8 6 3 7 1 4
Sorted Array:
1 2 3 4 5 6 7 8
```
在上面的代码中,`QuickSortAlgorithm` 函数递归地调用自身,直到排序完成。`Partition` 函数选择数组的最后一个元素作为主元(pivot),并将比主元小的元素交换到左侧,比主元大的元素交换到右侧,然后返回主元的下标。
`Swap` 函数用于交换数组中两个元素的值。
最后,`PrintArray` 函数将数组打印出来。
阅读全文
相关推荐
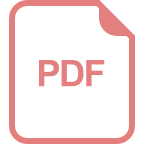
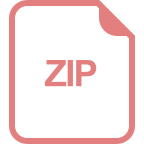















