好的,我可以为您提供一份基于element-ui二次封装的完整form表单代码,包括时间、select、input、checkbox、upload上传,并支持表单校验和自定义校验。只需要传递数据就可以自动渲染
时间: 2024-01-25 16:04:56 浏览: 82
好的,以下是基于element-ui二次封装的完整form表单代码,只需要传递数据就可以自动渲染:
Form.vue:
```html
<template>
<el-form ref="form" :model="formData" :rules="formRules" label-width="100px" style="max-width: 600px;">
<slot></slot>
<el-form-item>
<el-button type="primary" @click="submitForm">提交</el-button>
<el-button @click="resetForm">重置</el-button>
</el-form-item>
</el-form>
</template>
<script>
export default {
name: 'Form',
props: {
formData: {
type: Object,
default: () => ({})
},
formRules: {
type: Object,
default: () => ({})
}
},
methods: {
submitForm() {
this.$refs.form.validate((valid) => {
if (valid) {
this.$emit('submit', this.formData);
} else {
this.$message.error('表单校验失败,请检查表单信息');
return false;
}
});
},
resetForm() {
this.$refs.form.resetFields();
}
}
}
</script>
```
FormItem.vue:
```html
<template>
<el-form-item :label="label">
<component :is="type" v-model="formData[propName]" :options="options" :placeholder="placeholder" />
</el-form-item>
</template>
<script>
export default {
name: 'FormItem',
props: {
label: {
type: String,
default: ''
},
type: {
type: String,
default: 'input'
},
propName: {
type: String,
required: true
},
options: {
type: Array,
default: () => []
},
placeholder: {
type: String,
default: ''
}
},
inject: ['formData']
}
</script>
```
FormInput.vue:
```html
<template>
<el-input v-model="value" :placeholder="placeholder" />
</template>
<script>
export default {
name: 'FormInput',
props: {
value: {
type: String,
default: ''
},
placeholder: {
type: String,
default: ''
}
},
model: {
prop: 'value',
event: 'change'
},
methods: {
change(value) {
this.$emit('change', value);
}
}
}
</script>
```
FormSelect.vue:
```html
<template>
<el-select v-model="value" :placeholder="placeholder">
<el-option v-for="(option, index) in options" :key="index" :label="option.label" :value="option.value" />
</el-select>
</template>
<script>
export default {
name: 'FormSelect',
props: {
value: {
type: String,
default: ''
},
options: {
type: Array,
default: () => []
},
placeholder: {
type: String,
default: ''
}
},
model: {
prop: 'value',
event: 'change'
},
methods: {
change(value) {
this.$emit('change', value);
}
}
}
</script>
```
FormCheckbox.vue:
```html
<template>
<el-checkbox-group v-model="value">
<el-checkbox v-for="(option, index) in options" :key="index" :label="option.value">{{ option.label }}</el-checkbox>
</el-checkbox-group>
</template>
<script>
export default {
name: 'FormCheckbox',
props: {
value: {
type: Array,
default: () => []
},
options: {
type: Array,
default: () => []
}
},
model: {
prop: 'value',
event: 'change'
},
methods: {
change(value) {
this.$emit('change', value);
}
}
}
</script>
```
FormDateTime.vue:
```html
<template>
<el-date-picker v-model="value" type="datetime" :placeholder="placeholder" />
</template>
<script>
export default {
name: 'FormDateTime',
props: {
value: {
type: String,
default: ''
},
placeholder: {
type: String,
default: ''
}
},
model: {
prop: 'value',
event: 'change'
},
methods: {
change(value) {
this.$emit('change', value);
}
}
}
</script>
```
FormUpload.vue:
```html
<template>
<el-upload
class="upload-demo"
action="/upload"
:on-success="handleSuccess"
:on-error="handleError"
:before-upload="beforeUpload"
:file-list="fileList"
:limit="3"
:on-exceed="handleExceed"
:show-file-list="false">
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
</template>
<script>
export default {
name: 'FormUpload',
props: {
fileList: {
type: Array,
default: () => []
}
},
data() {
return {
uploadParam: {}
};
},
methods: {
handleSuccess(response, file, fileList) {
console.log(response, file, fileList);
},
handleError(error, file, fileList) {
console.log(error, file, fileList);
},
beforeUpload(file) {
const isJPG = file.type === 'image/jpeg';
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isJPG) {
this.$message.error('上传图片只能是 JPG 格式!');
}
if (!isLt2M) {
this.$message.error('上传图片大小不能超过 2MB!');
}
return isJPG && isLt2M;
},
handleExceed(files, fileList) {
this.$message.warning(`当前限制选择 3 个文件,本次选择了 ${files.length} 个文件,共选择了 ${files.length + fileList.length} 个文件`);
}
}
}
</script>
```
使用方法:
```html
<template>
<Form :formData="formData" :formRules="formRules" @submit="submitForm">
<FormItem label="时间" propName="time" type="FormDateTime" />
<FormItem label="选择" propName="select" type="FormSelect" :options="selectOptions" />
<FormItem label="输入" propName="input" type="FormInput" />
<FormItem label="多选框" propName="checkbox" type="FormCheckbox" :options="checkboxOptions" />
<FormItem propName="upload" type="FormUpload" :fileList="fileList" />
</Form>
</template>
<script>
import Form from '@/components/Form.vue';
import FormItem from '@/components/FormItem.vue';
import FormDateTime from '@/components/FormDateTime.vue';
import FormSelect from '@/components/FormSelect.vue';
import FormInput from '@/components/FormInput.vue';
import FormCheckbox from '@/components/FormCheckbox.vue';
import FormUpload from '@/components/FormUpload.vue';
export default {
components: {
Form,
FormItem,
FormDateTime,
FormSelect,
FormInput,
FormCheckbox,
FormUpload
},
data() {
return {
formData: {
time: '',
select: '',
input: '',
checkbox: [],
upload: ''
},
formRules: {
time: [{ required: true, message: '请选择时间', trigger: 'change' }],
select: [{ required: true, message: '请选择', trigger: 'change' }],
input: [{ required: true, message: '请输入', trigger: 'blur' }]
},
selectOptions: [
{ label: '选项1', value: 'option1' },
{ label: '选项2', value: 'option2' },
{ label: '选项3', value: 'option3' }
],
checkboxOptions: [
{ label: '选项1', value: 'option1' },
{ label: '选项2', value: 'option2' },
{ label: '选项3', value: 'option3' }
],
fileList: []
};
},
methods: {
submitForm(formData) {
console.log(formData);
this.$message.success('提交成功');
}
}
};
</script>
```
以上代码中,Form组件负责渲染整个表单,FormItem组件负责渲染表单项,所有表单项的数据都存储在formData中,表单校验规则存储在formRules中。
使用时,只需要在Form中传递formData和formRules,然后在FormItem中通过propName指定对应的数据,通过type指定渲染的组件类型,其他属性根据组件类型传递对应的属性即可。其中,FormInput、FormSelect、FormDateTime、FormCheckbox、FormUpload组件分别对应input、select、date-picker、checkbox-group和upload组件,同时支持v-model绑定和change事件。
希望这份代码能够满足您的需求。
阅读全文
相关推荐
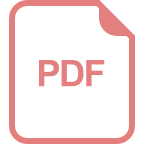
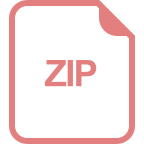

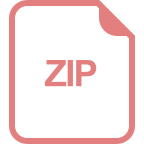
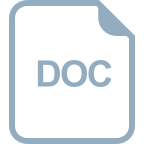
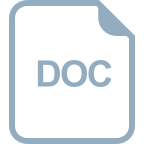
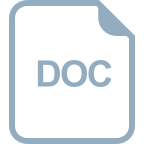


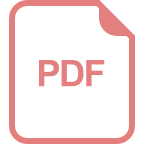
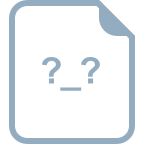
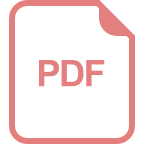
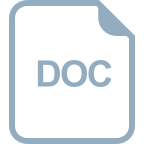
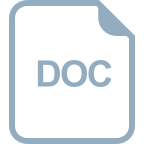
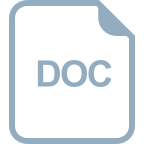
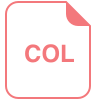
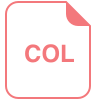
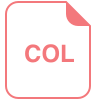