三维装箱算法matlab
时间: 2023-10-31 22:39:00 浏览: 54
三维装箱问题是一个经典的组合优化问题,其目标是将一批不同尺寸的立体物品尽可能地放入一个有限空间的立方体或长方体容器中,使得容器的体积最小。这个问题在物流、运输、仓库管理等领域有广泛的应用。
在MATLAB中实现三维装箱算法,可以采用以下步骤:
1. 确定立方体或长方体容器的尺寸和最大容积,以及需要装箱的物品的数量和尺寸。
2. 将物品按照体积从大到小排序,依次将每个物品放入容器中。
3. 对于每个物品,遍历容器中的所有位置,找到可以放置物品且剩余容积最小的位置。
4. 如果找到了合适的位置,则将物品放置在该位置,并更新容器剩余容积。
5. 如果找不到合适的位置,则扩大容器的尺寸,并将物品放置在新的位置上。
6. 重复步骤3-5,直到所有物品都被放置在容器中。
7. 输出容器尺寸和装箱方案。
需要注意的是,三维装箱问题是一个NP难问题,因此实现的算法可能无法找到全局最优解,只能得到一个近似解。
相关问题
三维装箱算法matlab,三维装箱遗传算法matlab程序
三维装箱算法和三维装箱遗传算法都是比较复杂的问题,需要一定的算法基础和编程能力才能完成。以下是一些可能有用的资源和参考代码:
1. 三维装箱问题的定义和解法可以参考维基百科的介绍:https://zh.wikipedia.org/wiki/三维装箱问题
2. 三维装箱问题的遗传算法解法可以参考这篇论文:https://www.researchgate.net/publication/324166915_Three-dimensional_packing_problem_using_genetic_algorithm
3. 三维装箱问题的 MATLAB 实现可以参考这个 GitHub 项目:https://github.com/nteczenek/3D-Bin-Packing
4. 如果你想自己编写三维装箱算法,可以参考这个博客的代码实现:https://blog.csdn.net/qq_39842530/article/details/107346865
需要注意的是,以上资源仅供参考,具体实现还需要根据自己的需求和实际情况进行调整。
贪心算法matlab三维装箱
贪心算法是一种常用的解决问题的方法,它通过每一步都选择当前最优的解决方案,从而达到整体最优的目标。在三维装箱问题中,我们需要将一系列物体放入一个三维容器中,同时要求最小化容器的体积。
在matlab中实现贪心算法解决三维装箱问题,可以按照以下步骤进行:
1. 首先,读取所有物体的尺寸信息,并根据体积进行排序,从大到小。
2. 创建一个三维容器,初始化容器的体积为0。
3. 从最大的物体开始,依次尝试将物体放入容器中。
4. 对于每个物体,遍历容器中已经放置的物体,找到一个位置使得物体的体积不与其他物体重叠,且能最大限度地利用容器空间。
5. 如果找到合适的位置,则将物体放入容器,并更新容器的体积。
6. 如果未找到合适的位置,则尝试放置下一个更小的物体。
7. 重复步骤4-6,直到所有物体都被放入容器中。
8. 输出容器的最终体积,即为贪心算法的解。
需要注意的是,贪心算法可能无法找到最优解,但可以得到一个较好的近似解。同时,该算法的效率也与排序的实现方式有关,可以选择合适的排序算法进行优化。
总之,通过使用贪心算法来解决三维装箱问题,可以在一定程度上最小化容器的体积,提高装箱效率。
相关推荐
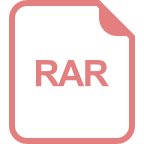





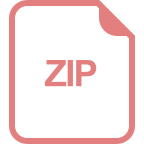
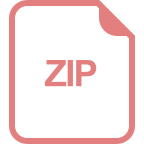
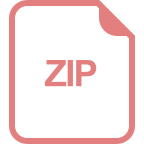
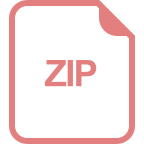
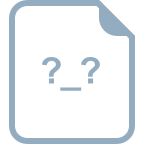
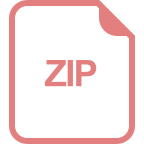
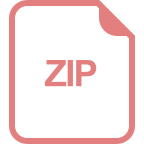