这是一个猜词游戏的规则说明,需要玩家或电脑根据设定的条件和提示来猜测正确的单词或组合。玩法包括猜单词和猜组合两种,猜单词需要猜出单词中的字母,猜组合需要猜出电脑设定的模式。游戏中有猜错次数限制和重复猜测限制,需要玩家或电脑在规定次数内猜出正确答案。每次猜测后,游戏都会显示当前猜出的残缺单词或猜测的组合情况,以及已经猜错的字母和剩余猜错次数。游戏的目标是在规定次数内猜出正确的单词或组合。用c++写出并表明思路
时间: 2023-12-06 17:44:54 浏览: 26
思路:
1. 首先需要定义一个包含所有待猜单词或组合的数组。
2. 然后需要设计随机选择单词或组合的函数。
3. 接着需要设计输入猜测的函数,用于读取玩家或电脑输入的猜测内容。
4. 设计判断猜测是否正确的函数,用于判断玩家或电脑的猜测是否与正确答案相同。
5. 定义猜错次数限制和重复猜测限制,当超过规定次数或重复猜测时,游戏结束。
6. 在每次猜测后,需要显示当前猜出的残缺单词或猜测的组合情况,以及已经猜错的字母和剩余猜错次数。
7. 游戏结束后,需要给出正确答案。
代码实现:
```c++
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAX_WRONG = 7; // 猜错次数上限
const string WORDS[] = {"APPLE", "ORANGE", "BANANA", "PEAR", "PEACH", "MANGO", "GRAPE", "WATERMELON", "STRAWBERRY", "BLUEBERRY"}; // 待猜单词数组
const int NUM_WORDS = sizeof(WORDS) / sizeof(string); // 待猜单词数量
int main()
{
srand(static_cast<unsigned int>(time(0))); // 随机数生成器的初始化
int choice = rand() % NUM_WORDS; // 随机选择一个待猜单词
string word = WORDS[choice]; // 正确答案
int wrong = 0; // 已经猜错的次数
string soFar(word.size(), '-'); // 残缺单词,初始状态为全“-”
string used = ""; // 已经猜过的字母
cout << "Welcome to the Word Jumble!\n\n";
cout << "Unscramble the letters to make a word.\n";
cout << "Enter 'quit' to quit the game.\n\n";
cout << "The jumble is: " << soFar << endl << endl;
while ((wrong < MAX_WRONG) && (soFar != word))
{
string guess;
cout << "Enter your guess: ";
cin >> guess;
if (guess == "quit")
break;
if (used.find(guess) != string::npos)
{
cout << "You've already guessed " << guess << endl;
continue;
}
used += guess;
if (word.find(guess) != string::npos)
{
cout << "That's right! " << guess << " is in the word.\n";
for (unsigned int i = 0; i < word.length(); ++i)
if (word[i] == guess)
soFar[i] = guess;
}
else
{
cout << "Sorry, " << guess << " is not in the word.\n";
++wrong;
}
cout << "The jumble is: " << soFar << endl;
cout << "Guesses so far: " << used << endl;
cout << "You have " << MAX_WRONG - wrong;
cout << " incorrect guesses left.\n\n";
}
if (soFar == word)
cout << "Congratulations! You guessed it!\n";
else
cout << "Sorry, you've used up all your guesses.\n";
cout << "The word was " << word << endl;
return 0;
}
```
以上是一个简单的猜单词游戏的代码实现。
相关推荐
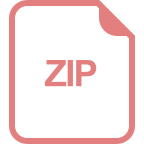
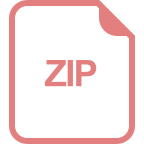














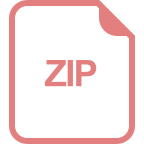