给我一个用lstm实现文本情感分析的案例的代码
时间: 2023-04-04 16:04:51 浏览: 78
以下是一个使用 LSTM 实现文本情感分析的案例代码:
```
import numpy as np
from keras.models import Sequential
from keras.layers import Dense, LSTM, Embedding
from keras.preprocessing.text import Tokenizer
from keras.preprocessing.sequence import pad_sequences
from keras.utils import to_categorical
# 定义数据集
texts = ['I love this movie', 'This movie is terrible', 'This is a great movie', 'I hate this movie']
labels = [1, 0, 1, 0]
# 对文本进行分词和编码
tokenizer = Tokenizer(num_words=1000)
tokenizer.fit_on_texts(texts)
sequences = tokenizer.texts_to_sequences(texts)
# 对序列进行填充
max_len = max([len(x) for x in sequences])
data = pad_sequences(sequences, maxlen=max_len)
# 将标签进行 one-hot 编码
labels = to_categorical(np.asarray(labels))
# 构建 LSTM 模型
model = Sequential()
model.add(Embedding(1000, 32, input_length=max_len))
model.add(LSTM(32))
model.add(Dense(2, activation='softmax'))
model.compile(optimizer='rmsprop', loss='categorical_crossentropy', metrics=['acc'])
# 训练模型
model.fit(data, labels, epochs=10, batch_size=32)
# 对新数据进行预测
new_texts = ['This is a fantastic movie', 'I do not like this film']
new_sequences = tokenizer.texts_to_sequences(new_texts)
new_data = pad_sequences(new_sequences, maxlen=max_len)
predictions = model.predict(new_data)
print(predictions)
```
这个代码使用 LSTM 模型对文本进行情感分析,将文本分为两类:正面和负面。模型使用 Embedding 层将文本编码为向量,然后使用 LSTM 层对向量进行处理,最后使用全连接层输出分类结果。
相关推荐
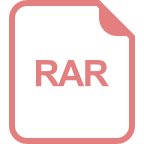
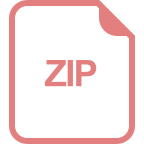














