如何让button水平向下居中对齐
时间: 2023-11-07 12:30:01 浏览: 36
要让Button控件水平向下居中对齐,可以使用RelativeLayout布局。具体实现方法如下:
```xml
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/my_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_alignParentBottom="true"
android:text="按钮"/>
</RelativeLayout>
```
其中,android:layout_centerHorizontal="true"表示让按钮水平居中,android:layout_alignParentBottom="true"表示让按钮在父布局的底部,即下方。这样,按钮就会水平向下居中对齐了。你可以根据需要进行调整。
相关问题
css让button水平居中
要让按钮水平居中,可以使用以下的 CSS 属性和值:
1. 将按钮元素的父元素设置为 flex 容器,并使用 flex 布局。
```css
.parent {
display: flex;
justify-content: center; /* 水平居中 */
align-items: center; /* 垂直居中,可选 */
}
```
2. 设置按钮元素的左右外边距为 auto。
```css
.button {
margin-left: auto;
margin-right: auto;
}
```
3. 将按钮元素的 display 属性设置为 inline-block。
```css
.button {
display: inline-block;
}
```
这样,按钮就会在其父元素中水平居中显示。请注意,父元素的宽度应该足够容纳按钮,否则按钮可能会换行显示。
两个div上下居中对齐
要实现两个div上下居中对齐,可以使用多种方法。其中一种方法是使用CSS中的flexbox布局。通过设置父容器的display属性为flex,然后使用align-items: center;属性来实现垂直居中对齐。这样,子元素就可以在父容器中上下居中对齐了。另一种方法是使用line-height属性。首先给父容器设置一个固定的高度,然后使用line-height属性,将其值设置为等于父容器的高度。这样,子元素就可以在父容器中上下居中对齐了。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [CSS 将两个button按钮垂直+水平居中](https://download.csdn.net/download/weixin_38723753/14887963)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [如何实现<div>中元素上下对齐](https://blog.csdn.net/memgxingfeixiang/article/details/52487359)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [在div中垂直居中的两个元素](https://blog.csdn.net/weixin_39853155/article/details/117878899)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
相关推荐
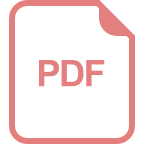
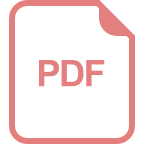
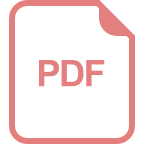












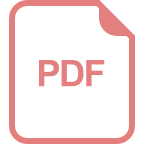