java转换excel生成json数据
时间: 2023-11-25 18:40:38 浏览: 43
可以使用 Apache POI 库来实现 Java 转换 Excel 生成 JSON 数据的功能。下面是一个简单的示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.json.JSONArray;
import org.json.JSONObject;
import java.io.FileInputStream;
import java.io.IOException;
public class ExcelToJsonConverter {
public static void main(String[] args) {
try {
FileInputStream fis = new FileInputStream("path_to_excel_file.xlsx");
Workbook workbook = new XSSFWorkbook(fis);
Sheet sheet = workbook.getSheetAt(0);
JSONArray jsonArray = new JSONArray();
for (Row row : sheet) {
JSONObject jsonObject = new JSONObject();
for (Cell cell : row) {
String columnName = sheet.getRow(0).getCell(cell.getColumnIndex()).getStringCellValue();
jsonObject.put(columnName, getCellValue(cell));
}
jsonArray.put(jsonObject);
}
System.out.println(jsonArray.toString());
workbook.close();
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static Object getCellValue(Cell cell) {
switch (cell.getCellType()) {
case STRING:
return cell.getStringCellValue();
case NUMERIC:
if (DateUtil.isCellDateFormatted(cell)) {
return cell.getDateCellValue();
} else {
return cell.getNumericCellValue();
}
case BOOLEAN:
return cell.getBooleanCellValue();
case FORMULA:
return cell.getCellFormula();
default:
return null;
}
}
}
```
请将 `path_to_excel_file.xlsx` 替换为实际的 Excel 文件路径。该示例将 Excel 文件中的数据读取并转换为 JSON 数组,并打印输出。你可以根据需要对输出进行进一步处理。
相关推荐
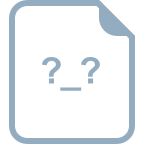










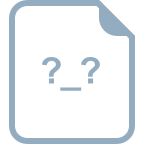
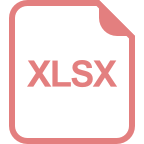
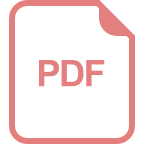