springboot调用接口获取下载地址并下载ofd文件
时间: 2023-05-31 19:05:17 浏览: 54
以下是一个简单的Spring Boot示例,调用接口获取下载地址并下载OFD文件:
1. 创建一个Spring Boot项目,并添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
```
2. 在`application.properties`中添加接口地址:
```
api.url=http://example.com/api/download
```
3. 创建一个`DownloadService`类,用于调用接口并下载OFD文件:
```
@Service
public class DownloadService {
@Value("${api.url}")
private String apiUrl;
public void download(String fileId, String filePath) throws IOException {
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost(apiUrl);
// 设置请求参数
List<NameValuePair> params = new ArrayList<>();
params.add(new BasicNameValuePair("fileId", fileId));
httpPost.setEntity(new UrlEncodedFormEntity(params));
// 发送请求
HttpResponse response = httpClient.execute(httpPost);
// 下载文件
HttpEntity entity = response.getEntity();
if (entity != null) {
BufferedInputStream bis = new BufferedInputStream(entity.getContent());
FileOutputStream fos = new FileOutputStream(filePath);
int inByte;
while ((inByte = bis.read()) != -1) {
fos.write(inByte);
}
bis.close();
fos.close();
}
}
}
```
4. 创建一个`DownloadController`类,用于接收请求并调用`DownloadService`:
```
@RestController
@RequestMapping("/download")
public class DownloadController {
@Autowired
private DownloadService downloadService;
@GetMapping("/{fileId}")
public ResponseEntity<?> download(@PathVariable("fileId") String fileId) {
try {
String filePath = "download/" + fileId + ".ofd";
downloadService.download(fileId, filePath);
File file = new File(filePath);
InputStreamResource resource = new InputStreamResource(new FileInputStream(file));
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Disposition", String.format("attachment; filename=\"%s\"", file.getName()));
headers.add("Cache-Control", "no-cache, no-store, must-revalidate");
headers.add("Pragma", "no-cache");
headers.add("Expires", "0");
return ResponseEntity.ok()
.headers(headers)
.contentLength(file.length())
.contentType(MediaType.parseMediaType("application/octet-stream"))
.body(resource);
} catch (Exception e) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(new ErrorResponse(e.getMessage()));
}
}
}
```
5. 运行项目并访问`http://localhost:8080/download/{fileId}`即可下载OFD文件。
相关推荐
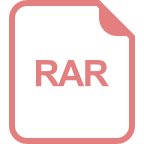














