在表单数据变化时,重新校验表单并更新校验提示消息,请给出完整代码示例
时间: 2024-02-21 07:59:54 浏览: 18
好的,以下是一个完整的uni-app表单校验示例,包括在表单数据变化时重新校验表单并更新校验提示消息的功能:
```vue
<template>
<view class="container">
<form ref="form">
<view class="form-item">
<text class="label">用户名:</text>
<input class="input" v-model="formData.username" />
</view>
<view class="form-item">
<text class="label">密码:</text>
<input class="input" type="password" v-model="formData.password" />
</view>
<view class="error-message">{{ errorMessage }}</view>
<view class="form-item">
<button class="submit-btn" @click="submitForm">提交</button>
</view>
</form>
</view>
</template>
<script>
export default {
data() {
return {
formData: {
username: '',
password: ''
},
errorMessage: ''
}
},
methods: {
// 提交表单
submitForm() {
// 校验表单
this.$refs.form.validate(valid => {
if (valid) {
// 表单校验通过,可以提交表单了
console.log('表单校验通过,可以提交表单了')
} else {
// 表单校验不通过,提示错误消息
this.errorMessage = '请正确输入表单'
}
})
}
},
watch: {
formData: {
deep: true,
handler: function (newVal) {
// 重新校验表单
this.$refs.form.validateField('username', err => {
if (err) {
// 更新校验提示消息
this.errorMessage = err.errors[0].message
} else {
this.errorMessage = ''
}
})
this.$refs.form.validateField('password', err => {
if (err) {
// 更新校验提示消息
this.errorMessage = err.errors[0].message
} else {
this.errorMessage = ''
}
})
}
}
}
}
</script>
<style>
.container {
display: flex;
justify-content: center;
align-items: center;
min-height: 100vh;
}
.form-item {
display: flex;
align-items: center;
margin-bottom: 20px;
}
.label {
width: 100px;
}
.input {
flex: 1;
height: 30px;
border: 1px solid #ccc;
border-radius: 3px;
padding: 0 10px;
}
.error-message {
color: red;
margin-bottom: 20px;
}
.submit-btn {
width: 200px;
height: 40px;
background-color: #409EFF;
color: #fff;
border: none;
border-radius: 3px;
cursor: pointer;
}
</style>
```
在该示例中,我们在watch监听器中监听了formData的变化,然后使用validateField方法重新校验表单,并在回调函数中更新了校验提示消息。这样,当用户在输入表单时,校验提示消息会实时更新,让用户更好地了解输入是否正确。当用户点击提交按钮时,会通过validate方法对整个表单进行校验,如果校验通过,则会提交表单。如果校验不通过,则会提示错误消息。
相关推荐
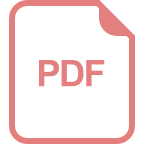
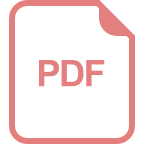
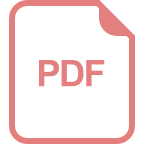














