react Fragment
时间: 2024-09-09 13:13:55 浏览: 50
在React中,`Fragment`是一个轻量级的元素,它不创建任何DOM元素,但可以用于组合多个元素,避免在JSX中直接使用`<></>`作为占位符。这有助于提高性能,因为`<></>`会被转换成真实DOM元素。以下是使用`Fragment`的示例:
```jsx
// 引入Fragment
import React, { Fragment } from "react";
// 定义App组件
class App extends React.Component {
render() {
// 使用Fragment包裹多个子元素
return (
<Fragment>
<h1>这是第一个元素</h1>
<p>这是第二个元素</p>
</Fragment>
);
}
}
// 注意:通常情况下,你不需要手动导入Fragment,因为它默认就在React的顶层API中
// 如果你想明确指定版本,则可以导入,如上面所示
```
`Fragment`的主要作用在于组织逻辑,而不是改变组件的行为。正如引用所说[^1],它就像一个"容器",让你可以在不创建多余DOM节点的情况下组合元素。
相关问题
Invalid prop `authority` supplied to `React.Fragment`. React.Fragment can only have `key` and `children` props.
这个错误提示是因为React.Fragment组件的props只能包含`key`和`children`,而你给它传递了一个`authority`属性。请检查你的代码,找到并删除这个错误的属性。你可以参考React.Fragment的文档来了解更多关于这个组件的使用方法:https://zh-hans.reactjs.org/docs/fragments.html
react常见面试题
React常见面试题包括但不限于以下几个方面:
1. React组件的生命周期:React组件的生命周期分为三个阶段,分别是挂载阶段、更新阶段和卸载阶段。在每个阶段,都有相应的生命周期方法可以被调用,比如componentDidMount、componentDidUpdate和componentWillUnmount等。
2. React的虚拟DOM和DOM diff算法:React通过使用虚拟DOM来提高性能。虚拟DOM是一个轻量级的JavaScript对象,它表示真实DOM的层次结构。当数据发生变化时,React会使用DOM diff算法来比较新旧虚拟DOM树的差异,并只更新需要变化的部分,从而避免了对整个DOM树进行重渲染。
3. React中的状态管理:React有多种状态管理的方式,最常用的是使用组件的state和props来管理状态。另外,还可以使用React的Context API、Redux、Mobx等来实现更灵活的状态管理。
4. React中的事件处理:在React中,可以通过给元素添加事件处理函数来处理用户的交互行为。常见的事件处理方式包括直接在JSX中绑定事件处理函数、使用类组件中的成员方法作为事件处理函数、使用React的Hooks中的useEffect来处理副作用等。
5. React的组件通信:React组件之间的通信可以通过props传递数据、使用React的Context API、使用全局状态管理工具等方式实现。另外,如果组件之间的通信比较复杂,还可以考虑使用消息订阅-发布模式或者事件总线来实现。
6. React的性能优化:React提供了一些性能优化的手段,比如使用shouldComponentUpdate来控制组件的更新、使用React的memo和useMemo来缓存计算结果、使用React的Fragment来减少不必要的DOM节点等。
相关推荐
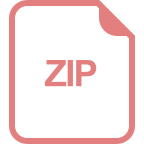
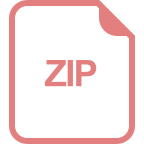
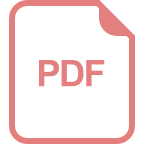
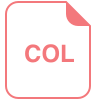











