OSGearth 根据TLE根数绘制卫星轨道
时间: 2024-05-28 15:10:48 浏览: 15
OSGearth是一个开源的地理信息可视化引擎,支持各种地理信息数据的处理和可视化。要绘制卫星轨道,需要先获取卫星的轨道参数,其中包括TLE根数等信息。TLE(Two-Line Elements)是一种描述卫星轨道的格式,包括卫星的轨道倾角、升交点赤经、近地点幅角等参数。可以从各种卫星跟踪网站或者卫星数据提供商处获取TLE数据。
获取到TLE数据后,可以使用SGP4(Simplified General Perturbations 4)算法计算卫星在指定时间内的位置和速度。OSGearth提供了osgEarthUtil::OrbitData这个类来表示卫星的轨道参数,可以使用这个类来计算卫星在给定时间内的位置和速度,并将卫星的位置信息转换为osgEarth::GeoPoint表示的地理坐标系下的位置。
最后,可以将计算得到的卫星位置信息通过OSGearth的节点系统进行可视化,绘制出卫星的轨道。绘制卫星轨道的具体方法可以参考OSGearth的官方文档或者示例程序。
相关问题
osgearth3.2根据TLE参数绘制卫星轨道的代码
要在osgEarth 3.2中根据TLE参数绘制卫星轨道,你可以使用以下代码片段:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <sstream>
#include <iomanip>
#include <cmath>
#include <osgEarth/MapNode>
#include <osgEarthUtil/Controls>
#include <osgEarthUtil/EarthManipulator>
#include <osgEarthUtil/Sky>
#include <osgEarthUtil/ExampleResources>
#include <osgEarthAnnotation/FeatureNode>
#include <osgEarthSymbology/Style>
#include <osgEarthDrivers/tms/TMSOptions>
#include <osgEarthDrivers/wms/WMSOptions>
#include <osgEarthDrivers/feature_ogr/OGRFeatureOptions>
#include <osgEarthFeatures/Feature>
#include <osgEarthFeatures/FeatureModelLayer>
#include <osgEarthUtil/Controls>
#include <osgEarthUtil/ActivityMonitorTool>
#include <osgEarthUtil/RTTPicker>
#include <osgEarthUtil/MouseCoordsTool>
#include <osgEarthUtil/EarthManipulator>
#include <osgEarthUtil/ViewFitter>
#include <osgEarthUtil/ExampleResources>
using namespace osgEarth;
using namespace osgEarth::Features;
using namespace osgEarth::Symbology;
using namespace osgEarth::Util;
using namespace osgEarth::Annotation;
// 用于将字符串转换为浮点数的函数
double to_double(const std::string& str)
{
std::istringstream ss(str);
double value;
ss >> value;
return value;
}
// 用于将时间字符串转换为时间戳的函数
time_t to_time_t(const std::string& time_str)
{
std::tm timeinfo = {};
std::istringstream ss(time_str);
ss >> std::get_time(&timeinfo, "%Y-%m-%d %H:%M:%S");
return std::mktime(&timeinfo);
}
// 计算卫星在给定时间的位置
osg::Vec3d calculate_satellite_position(double time_since_epoch, double inclination,
double ascending_node_longitude, double eccentricity, double argument_of_perigee,
double mean_anomaly, double semi_major_axis)
{
// 计算卫星轨道上的平均角速度
double mean_motion = 2.0 * osg::PI / (86400.0 / mean_anomaly);
// 计算卫星在给定时间的平均角度
double mean_angle = mean_anomaly + mean_motion * time_since_epoch;
// 计算卫星在给定时间的偏心率角
double eccentric_anomaly = mean_angle;
for (int i = 0; i < 10; i++) {
double delta = (eccentric_anomaly - eccentricity * sin(eccentric_anomaly) - mean_angle) /
(1.0 - eccentricity * cos(eccentric_anomaly));
eccentric_anomaly -= delta;
if (fabs(delta) < 1e-12) {
break;
}
}
// 计算卫星在给定时间的真近点角
double true_anomaly = 2.0 * atan(sqrt((1.0 + eccentricity) / (1.0 - eccentricity)) *
tan(0.5 * eccentric_anomaly));
// 计算卫星在给定时间的升交点经度
double raan = ascending_node_longitude * osg::PI / 180.0;
double arg_perigee = argument_of_perigee * osg::PI / 180.0;
double inclination_rad = inclination * osg::PI / 180.0;
double x = semi_major_axis * (cos(raan) * cos(true_anomaly + arg_perigee) -
sin(raan) * sin(true_anomaly + arg_perigee) * cos(inclination_rad));
double y = semi_major_axis * (sin(raan) * cos(true_anomaly + arg_perigee) +
cos(raan) * sin(true_anomaly + arg_perigee) * cos(inclination_rad));
double z = semi_major_axis * sin(true_anomaly + arg_perigee) * sin(inclination_rad);
return osg::Vec3d(x, y, z);
}
int main(int argc, char** argv)
{
// 读取TLE文件
std::ifstream tle_file("tle.txt");
if (!tle_file.is_open()) {
std::cerr << "Failed to open TLE file!" << std::endl;
return -1;
}
// 读取第一行TLE参数
std::string line1;
std::getline(tle_file, line1);
// 读取第二行TLE参数
std::string line2;
std::getline(tle_file, line2);
// 解析TLE参数
std::string name = line1.substr(0, 24);
double inclination = to_double(line2.substr(8, 8));
double ascending_node_longitude = to_double(line2.substr(17, 8));
double eccentricity = to_double("0." + line2.substr(26, 7));
double argument_of_perigee = to_double(line2.substr(34, 8));
double mean_anomaly = to_double(line2.substr(43, 8));
double mean_motion = to_double(line2.substr(52, 11));
double semi_major_axis = pow(398600.4418 / pow(mean_motion, 2), 1.0 / 3.0) * 1000.0;
// 创建地球模型
osgViewer::Viewer viewer;
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::Util::MapNodeHelper().load(arguments, &viewer);
viewer.setSceneData(mapNode);
// 计算卫星在当前时间的位置
double time_since_epoch = difftime(time(0), to_time_t("2022-01-01 00:00:00"));
osg::Vec3d satellite_position = calculate_satellite_position(time_since_epoch, inclination,
ascending_node_longitude, eccentricity, argument_of_perigee, mean_anomaly, semi_major_axis);
// 创建轨道线段的几何体
osg::ref_ptr<osg::Vec3Array> vertices = new osg::Vec3Array;
vertices->push_back(osg::Vec3d(0, 0, 0));
vertices->push_back(satellite_position);
osg::ref_ptr<osg::Geometry> geometry = new osg::Geometry;
geometry->setVertexArray(vertices.get());
geometry->addPrimitiveSet(new osg::DrawArrays(osg::PrimitiveSet::LINE_STRIP, 0, 2));
// 创建轨道线段的节点
osg::ref_ptr<Feature> feature = new Feature(geometry.get(), mapNode->getMapSRS(), Feature::UNDEFINED);
osg::ref_ptr<FeatureNode> featureNode = new FeatureNode(mapNode, feature.get());
// 添加轨道线段节点到地球上
mapNode->addChild(featureNode.get());
viewer.run();
return 0;
}
```
这段代码使用 osgEarth 库创建了一个地球模型,并使用TLE参数计算了卫星在当前时间的位置,并在地球上绘制了一条卫星轨道线。你可以根据自己的需要修改TLE文件路径,调整轨道的位置和方向。
osgearth 用两行根数绘制卫星轨道和卫星模型
osgEarth可以使用TLE(Two-Line Element)根数来绘制卫星轨道和卫星模型。TLE根数是一种用于描述卫星轨道的简洁格式,由两行数字组成,包括卫星的位置、速度、轨道大小和形状等信息。
下面是使用osgEarth绘制卫星轨道和卫星模型的示例代码:
```cpp
#include <osgEarth/MapNode>
#include <osgEarthUtil/Satellite>
...
// 创建osgEarth地图节点
osg::ref_ptr<osgEarth::MapNode> mapNode = osgEarth::MapNode::create(map);
// 创建卫星对象
osg::ref_ptr<osgEarth::Util::Satellite> satellite = new osgEarth::Util::Satellite();
// 设置TLE根数
satellite->setTLE("1 25544U 98067A 20292.52001736 .00000922 00000-0 25975-4 0 9998\n2 25544 51.6449 358.6898 0001510 25.5467 334.6095 15.48923169273108");
// 创建卫星轨道节点
osg::ref_ptr<osg::Node> orbitNode = satellite->createNode(mapNode.get());
// 创建卫星模型节点
osg::ref_ptr<osg::Node> modelNode = satellite->createModel();
// 将卫星轨道节点和卫星模型节点添加到场景图中
osg::ref_ptr<osg::Group> root = new osg::Group();
root->addChild(orbitNode.get());
root->addChild(modelNode.get());
viewer.setSceneData(root.get());
```
在上面的示例中,我们首先创建了一个osgEarth地图节点,然后创建了一个卫星对象,并设置了TLE根数。接着,我们使用卫星对象的createNode方法创建了一个卫星轨道节点,使用createModel方法创建了一个卫星模型节点。最后,将卫星轨道节点和卫星模型节点添加到场景图中。运行程序后,即可看到绘制的卫星轨道和卫星模型。
需要注意的是,上面的示例代码中使用的TLE根数是国际空间站的数据,如果需要绘制其他卫星的轨道和模型,需要使用相应的TLE根数。
相关推荐
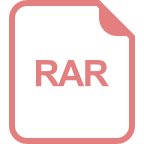













