python查找指定字符位置
时间: 2023-11-27 08:06:25 浏览: 67
你可以使用字符串的 `find()` 方法来查找指定字符的位置,该方法返回该字符在字符串中第一次出现的下标。例如,如果你想查找字符 `a` 在字符串 `hello world` 中的位置,可以使用以下代码:
```
s = "hello world"
index = s.find('a')
print(index)
```
输出结果为:
```
-1
```
如果字符 `a` 在字符串中不存在,`find()` 方法会返回 `-1`。如果字符存在,则返回该字符在字符串中的下标,下标从 `0` 开始计数。
相关问题
python查找指定字符
可以使用Python中的字符串方法`find()`或`index()`来查找指定字符在字符串中的位置。
`find()`方法会从左往右查找指定字符,并返回它在字符串中的第一个出现位置的索引值。如果指定字符不存在,则返回-1。
例如,查找字符串s中字符'a'的位置:
```python
s = 'hello world'
pos = s.find('a')
print(pos) # 输出: -1
```
`index()`方法与`find()`类似,但是如果指定字符不存在,则会抛出ValueError异常。
例如,查找字符串s中字符'o'的位置:
```python
s = 'hello world'
pos = s.index('o')
print(pos) # 输出: 4
```
如果要查找字符串中所有指定字符的位置,则可以使用列表推导式:
```python
s = 'hello world'
char = 'l'
pos = [i for i, c in enumerate(s) if c == char]
print(pos) # 输出: [2, 3, 9]
```
以上代码使用了字符串方法`enumerate()`,它返回一个枚举对象,包含字符串中字符的索引和字符本身。列表推导式遍历枚举对象,并将所有字符为指定字符的索引保存到列表中。
python 查找指定字符方法
在 Python 中查找指定字符(或子串)的方法很多,下面列举几种常见的方法:
1. 使用 in 操作符:可以使用 in 操作符来判断一个字符(或子串)是否在另一个字符串中出现过。例如:
```python
s = 'hello world'
if 'o' in s:
print('o exists in s')
```
2. 使用 find() 方法:可以使用 find() 方法来查找一个字符(或子串)在另一个字符串中的位置,如果找不到,则返回 -1。例如:
```python
s = 'hello world'
index = s.find('o')
if index != -1:
print('o exists in s at index', index)
```
3. 使用 index() 方法:与 find() 方法类似,但是如果找不到指定字符(或子串),则会抛出 ValueError 异常。例如:
```python
s = 'hello world'
index = s.index('o')
print('o exists in s at index', index)
```
4. 使用 count() 方法:可以使用 count() 方法来统计一个字符(或子串)在另一个字符串中出现的次数。例如:
```python
s = 'hello world'
count = s.count('o')
print('o appears', count, 'times in s')
```
以上是常见的几种方法,你可以根据自己的需求选择适合自己的方法来查找指定字符(或子串)。
阅读全文
相关推荐











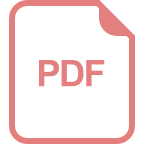