请你帮我编写一个基于Java的MES系统
时间: 2024-01-24 14:20:46 浏览: 175
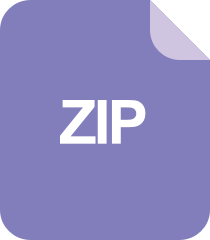
基于Java的MES生产管理系统源码.zip
MES (Manufacturing Execution System) 是一种用于控制制造过程的计算机系统。它通常包括以下模块:生产调度、物料跟踪、质量控制、人力资源管理等。下面是一个简单的MES系统的Java实现,供你参考。
1. 生产调度模块
```java
public class ProductionScheduler {
private List<Job> jobList;
private MachineManager machineManager;
public ProductionScheduler(List<Job> jobList, MachineManager machineManager) {
this.jobList = jobList;
this.machineManager = machineManager;
}
public void schedule() {
for (Job job : jobList) {
Machine machine = machineManager.getAvailableMachine(job.getMachineType());
if (machine == null) {
System.out.println("No available machine for job " + job.getId());
continue;
}
machine.startJob(job);
}
}
}
```
2. 物料跟踪模块
```java
public class MaterialTracker {
private Map<String, Integer> inventory;
public MaterialTracker(Map<String, Integer> inventory) {
this.inventory = inventory;
}
public boolean consumeMaterials(Job job) {
Map<String, Integer> requiredMaterials = job.getRequiredMaterials();
for (Map.Entry<String, Integer> entry : requiredMaterials.entrySet()) {
String materialName = entry.getKey();
int requiredQuantity = entry.getValue();
if (inventory.getOrDefault(materialName, 0) < requiredQuantity) {
System.out.println("Insufficient " + materialName + " for job " + job.getId());
return false;
}
}
for (Map.Entry<String, Integer> entry : requiredMaterials.entrySet()) {
String materialName = entry.getKey();
int requiredQuantity = entry.getValue();
inventory.put(materialName, inventory.getOrDefault(materialName, 0) - requiredQuantity);
}
return true;
}
public void addInventory(String materialName, int quantity) {
inventory.put(materialName, inventory.getOrDefault(materialName, 0) + quantity);
}
}
```
3. 质量控制模块
```java
public class QualityController {
private Map<String, Integer> defectCounts;
public QualityController() {
this.defectCounts = new HashMap<>();
}
public boolean inspect(Product product) {
boolean isDefective = // perform quality inspection
if (isDefective) {
String defectType = // determine defect type
defectCounts.put(defectType, defectCounts.getOrDefault(defectType, 0) + 1);
}
return !isDefective;
}
public Map<String, Integer> getDefectCounts() {
return defectCounts;
}
}
```
4. 人力资源管理模块
```java
public class HumanResourceManager {
private List<Employee> employeeList;
public HumanResourceManager(List<Employee> employeeList) {
this.employeeList = employeeList;
}
public Employee getAvailableEmployee(String skill) {
for (Employee employee : employeeList) {
if (employee.hasSkill(skill) && !employee.isBusy()) {
return employee;
}
}
return null;
}
}
```
5. MES系统主类
```java
public class MESSystem {
public static void main(String[] args) {
// initialize data
List<Job> jobList = // load job data
MachineManager machineManager = // create machine manager
MaterialTracker materialTracker = // create material tracker
QualityController qualityController = // create quality controller
HumanResourceManager humanResourceManager = // create human resource manager
// create production scheduler
ProductionScheduler productionScheduler = new ProductionScheduler(jobList, machineManager);
// start production
productionScheduler.schedule();
// inspect products
int numDefectiveProducts = 0;
for (Product product : machineManager.getFinishedProducts()) {
if (!qualityController.inspect(product)) {
numDefectiveProducts++;
}
}
System.out.println("Defect types and counts: " + qualityController.getDefectCounts());
// log output
// ...
}
}
```
以上代码只是一个简单的MES系统的Java实现,具体实现还需要根据实际情况进行调整和完善。
阅读全文
相关推荐

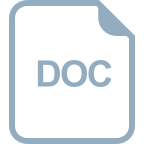
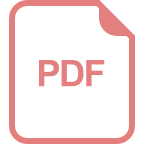
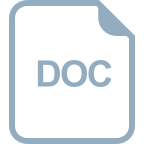
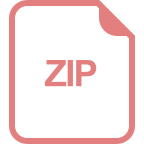
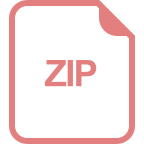
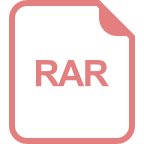
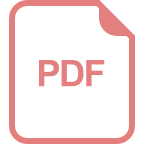
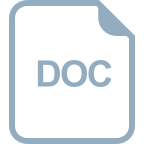
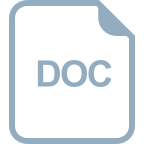
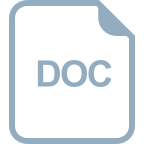
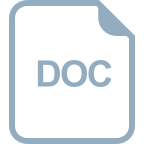
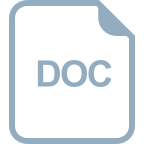
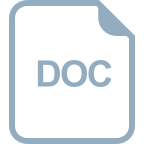
