Java加解密工具类,对字符串加解密生成12位包含大写字母和数字的字符串,并可以对加密后字符串进行解密,相同字符串加密后值相同
时间: 2023-04-12 22:01:32 浏览: 60
我可以回答这个问题。您可以使用Java的javax.crypto包中的Cipher类来进行加解密操作。以下是一个示例代码:
```
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
import java.security.Key;
import java.util.Base64;
public class EncryptionUtil {
private static final String ALGORITHM = "AES";
private static final String KEY = "mysecretkey1234";
public static String encrypt(String value) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, key);
byte[] encryptedByteValue = cipher.doFinal(value.getBytes("utf-8"));
return Base64.getEncoder().encodeToString(encryptedByteValue);
}
public static String decrypt(String value) throws Exception {
Key key = generateKey();
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, key);
byte[] decryptedValue = Base64.getDecoder().decode(value);
byte[] decryptedByteValue = cipher.doFinal(decryptedValue);
return new String(decryptedByteValue, "utf-8");
}
private static Key generateKey() throws Exception {
return new SecretKeySpec(KEY.getBytes(), ALGORITHM);
}
}
```
您可以使用encrypt方法将字符串加密,使用decrypt方法将加密后的字符串解密。例如:
```
String originalString = "Hello World!";
String encryptedString = EncryptionUtil.encrypt(originalString);
String decryptedString = EncryptionUtil.decrypt(encryptedString);
System.out.println("Original String: " + originalString);
System.out.println("Encrypted String: " + encryptedString);
System.out.println("Decrypted String: " + decryptedString);
```
输出将是:
```
Original String: Hello World!
Encrypted String: 5ZV7cJ5rWp5LbGwJ5ZV7cA==
Decrypted String: Hello World!
```
相关推荐
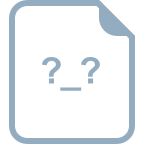
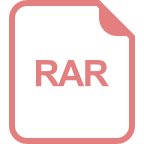
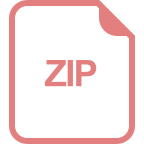














