vue如何实现文件上传
时间: 2024-01-31 19:02:06 浏览: 48
Vue可以通过使用第三方库或者自己手写上传组件来实现文件上传。最常用的第三方库是vue-uploader和vue-file-upload。手写上传组件需要使用HTML5的FormData对象来构造上传请求,然后使用XMLHttpRequest对象来发送请求。可以通过监听XMLHttpRequest对象的progress事件来显示上传进度。
相关问题
springboot VUE实现文件上传
如何实现SpringBoot+Vue文件上传?
文件上传涉及前端和后端两个方面的实现。
前端的Vue代码:
1. 定义上传文件的模板:
```
<template>
<div>
<input type="file" @change="handleFileUpload" ref="fileUpload">
<button @click="submitFile">上传文件</button>
</div>
</template>
```
2. 在Vue的methods中添加上传文件的方法:
```
methods: {
handleFileUpload () {
this.file = this.$refs.fileUpload.files[0]
},
submitFile () {
let formData = new FormData()
formData.append('file', this.file)
axios.post('/api/upload', formData, {
headers: {
'Content-Type': 'multipart/form-data'
}
})
.then(response => {
console.log(response.data)
})
}
}
```
这个方法中,我们通过FormData对象来将文件对象上传到服务器端。需要注意的是,在axios请求中,我们需要指定Content-Type为multipart/form-data,以便后端能够正确地解析上传的文件。
后端的SpringBoot代码:
1. 配置文件上传的Multipart配置
在application.properties文件中添加以下配置:
```
spring.servlet.multipart.max-file-size=10MB
spring.servlet.multipart.max-request-size=10MB
```
这个配置指定了上传文件的大小限制,例如,上限设置为10MB。
2. 添加文件上传的Controller
```
@RestController
@RequestMapping("/api")
public class FileUploadController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
// 将上传的文件保存到指定路径下
String filePath = "C:/uploads/" + file.getOriginalFilename();
file.transferTo(new File(filePath));
return "文件上传成功";
} catch (IOException e) {
e.printStackTrace();
return "文件上传失败";
}
}
}
```
这个Controller中,通过@RequestParam注解来指定上传的文件参数名,再通过MultipartFile来获取上传的文件。最后,将文件保存到指定的路径下。需要注意的是,保存路径需要在业务中合理设置。
至此,SpringBoot+Vue文件上传的实现就完成了。
vue实现文件上传和下载
文件上传和下载是前端开发中非常常见的功能,Vue提供了方便的方法来实现这些功能。
文件上传:
Vue中可以使用第三方库vue-file-upload来实现文件上传,具体步骤如下:
1. 安装vue-file-upload
```sh
npm install vue-file-upload --save
```
2. 在组件中引入并注册vue-file-upload
```js
import Vue from 'vue';
import VueFileUpload from 'vue-file-upload';
Vue.use(VueFileUpload);
```
3. 在模板中使用vue-file-upload来实现文件上传
```html
<template>
<div>
<vue-file-upload
url="/api/upload"
@success="onUploadSuccess"
@error="onUploadError"
@progress="onUploadProgress"
></vue-file-upload>
</div>
</template>
```
其中,url属性指定上传文件的地址,success、error和progress分别指定上传成功、上传失败和上传进度的回调函数。
文件下载:
Vue中可以使用原生的XMLHttpRequest对象或者第三方库axios来实现文件下载,具体步骤如下:
1. 使用XMLHttpRequest对象下载文件
```js
const xhr = new XMLHttpRequest();
xhr.open('GET', '/api/download', true);
xhr.responseType = 'blob';
xhr.onload = () => {
if (xhr.status === 200) {
const blob = new Blob([xhr.response], { type: 'application/octet-stream' });
const url = URL.createObjectURL(blob);
const link = document.createElement('a');
link.href = url;
link.download = 'file.txt';
link.click();
URL.revokeObjectURL(url);
}
};
xhr.send();
```
其中,responseType属性指定响应类型为blob,onload事件中将响应数据转为Blob对象,并创建一个超链接来下载文件。
2. 使用axios下载文件
```js
axios({
method: 'get',
url: '/api/download',
responseType: 'blob',
}).then((response) => {
const blob = new Blob([response.data], { type: 'application/octet-stream' });
const url = URL.createObjectURL(blob);
const link = document.createElement('a');
link.href = url;
link.download = 'file.txt';
link.click();
URL.revokeObjectURL(url);
});
```
其中,responseType属性指定响应类型为blob,然后将响应数据转为Blob对象,并创建一个超链接来下载文件。
阅读全文
相关推荐
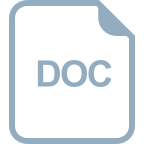
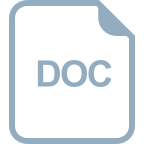
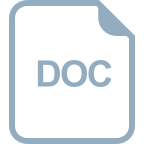
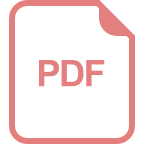

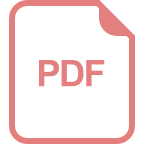
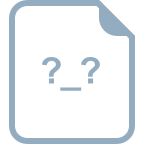
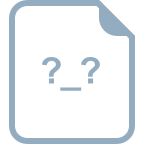
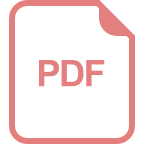
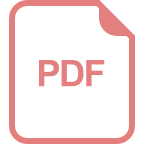






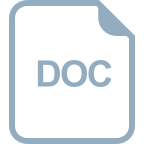