用c++分支限界法写出算法捕牛记问题
时间: 2024-05-12 21:16:47 浏览: 98
好的,针对您的问题,下面是使用 C 分支限界法求解捕牛记问题的算法:
首先,我们需要定义一个结构体来存储牛、人和草的状态:
```c
struct State {
int cow1; // 第一头牛的位置
int cow2; // 第二头牛的位置
int person; // 人的位置
int grass; // 草的位置
int cost; // 到达当前状态的步数
};
```
接下来,我们使用一个优先队列(堆)来存储待扩展的状态。每次从队列中取出步数最小的状态进行扩展,直到找到目标状态为止。
```c
int target_cow1, target_cow2, target_person, target_grass;
// 比较函数,用于定义优先队列中元素的顺序
bool operator<(const State& a, const State& b) {
return a.cost > b.cost;
}
int bfs() {
priority_queue<State> pq;
pq.push({1, 2, 3, 4, 0});
while (!pq.empty()) {
State curr = pq.top();
pq.pop();
// 如果当前状态与目标状态相同,则返回步数
if (curr.cow1 == target_cow1 && curr.cow2 == target_cow2 &&
curr.person == target_person && curr.grass == target_grass) {
return curr.cost;
}
// 扩展当前状态
for (int i = 1; i <= 4; i++) {
// 移动人到下一个位置
State next;
next.cow1 = curr.cow1;
next.cow2 = curr.cow2;
next.person = i;
next.grass = curr.grass;
next.cost = curr.cost + 1;
// 判断人是否被牛吃掉
if (curr.person == curr.cow1 || curr.person == curr.cow2 || i == curr.cow1 || i == curr.cow2) {
continue;
}
// 判断人是否吃到草
if (i == curr.grass) {
next.grass = 0;
}
// 移动第一头牛
if (curr.person == curr.cow1) {
if (i == curr.cow2) {
continue;
}
next.cow1 = i;
}
// 移动第二头牛
if (curr.person == curr.cow2) {
if (i == curr.cow1) {
continue;
}
next.cow2 = i;
}
// 加入优先队列
pq.push(next);
}
}
// 如果无法到达目标状态,则返回 -1
return -1;
}
```
在主函数中,我们可以输入目标状态,调用 bfs() 函数来求解最少需要多少步才能达到目标状态。
```c
int main() {
cin >> target_cow1 >> target_cow2 >> target_person >> target_grass;
int steps = bfs();
cout << steps << endl;
return 0;
}
```
希望这个算法能够帮助到您,如果您还有其他问题或者疑惑,请随时提出,我会尽力为您解答。
相关推荐
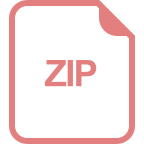
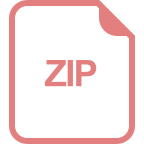
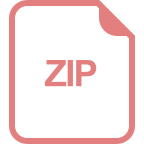
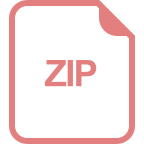
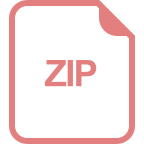
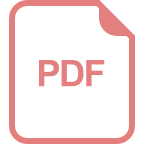
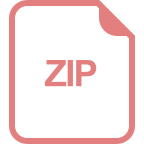
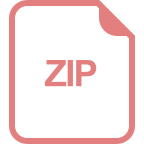
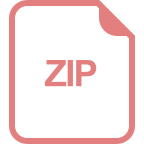
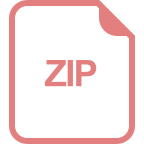