C语言复制文件夹代码Windows版
时间: 2024-04-23 16:01:57 浏览: 201
要在C语言中复制文件夹,您可以使用Windows API中的一些函数来执行此操作。以下是一个示例代码,可以在Windows上复制文件夹:
```c
#include <stdio.h>
#include <stdbool.h>
#include <windows.h>
bool CopyDirectory(const char* srcPath, const char* destPath) {
// 创建目标文件夹
if (!CreateDirectory(destPath, NULL)) {
fprintf(stderr, "Failed to create destination directory.\n");
return false;
}
char srcFile[MAX_PATH];
char destFile[MAX_PATH];
WIN32_FIND_DATA findData;
// 构建源文件夹路径
snprintf(srcFile, sizeof(srcFile), "%s\\*", srcPath);
// 查找源文件夹中的所有文件和子文件夹
HANDLE hFind = FindFirstFile(srcFile, &findData);
if (hFind == INVALID_HANDLE_VALUE) {
fprintf(stderr, "Failed to find first file.\n");
return false;
}
do {
if (strcmp(findData.cFileName, ".") != 0 && strcmp(findData.cFileName, "..") != 0) {
// 构建源文件/文件夹路径
snprintf(srcFile, sizeof(srcFile), "%s\\%s", srcPath, findData.cFileName);
// 构建目标文件/文件夹路径
snprintf(destFile, sizeof(destFile), "%s\\%s", destPath, findData.cFileName);
if (findData.dwFileAttributes & FILE_ATTRIBUTE_DIRECTORY) {
// 如果是子文件夹,则递归调用CopyDirectory函数复制子文件夹
if (!CopyDirectory(srcFile, destFile)) {
FindClose(hFind);
return false;
}
} else {
// 如果是文件,则复制文件
if (!CopyFile(srcFile, destFile, FALSE)) {
fprintf(stderr, "Failed to copy file: %s\n", srcFile);
FindClose(hFind);
return false;
}
}
}
} while (FindNextFile(hFind, &findData));
FindClose(hFind);
return true;
}
int main() {
const char* srcPath = "C:\\Path\\To\\Source";
const char* destPath = "C:\\Path\\To\\Destination";
if (CopyDirectory(srcPath, destPath)) {
printf("Directory copied successfully.\n");
} else {
fprintf(stderr, "Failed to copy directory.\n");
}
return 0;
}
```
在上面的示例中,`CopyDirectory`函数通过递归地遍历源文件夹中的所有文件和子文件夹,并使用`CopyFile`函数复制文件,使用`CreateDirectory`函数创建新的文件夹。请注意,`CopyDirectory`函数在复制文件时不会保留文件的元数据(例如权限等),仅复制文件的内容。
在使用示例代码之前,请将源路径和目标路径替换为您要复制的实际路径。
希望对您有所帮助!如有任何其他问题,请随时提问。
阅读全文
相关推荐


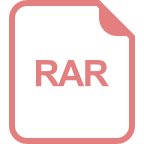
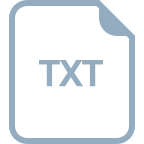
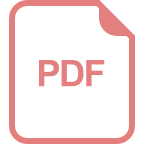
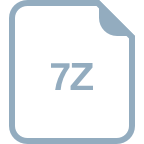
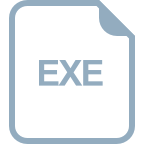
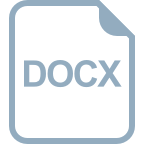
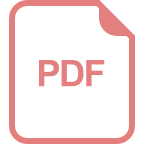
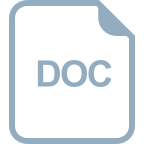
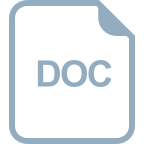
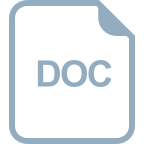
