FIFO LRU python
时间: 2023-11-08 08:03:41 浏览: 60
FIFO(First-In-First-Out)和LRU(Least Recently Used)都是常用的缓存淘汰算法。它们用于在缓存空间满时确定要被替换的元素。
FIFO算法按照元素进入缓存的顺序进行淘汰。当缓存空间满时,最先进入缓存的元素将被替换掉。
LRU算法根据元素的使用频率进行淘汰。当缓存空间满时,最近最少使用的元素将被替换掉。
下面是使用Python实现FIFO和LRU算法的示例代码:
FIFO算法示例代码:
```python
class FIFO:
def __init__(self, capacity):
self.capacity = capacity
self.cache = []
def get(self, key):
if key in self.cache:
return key
else:
return -1
def put(self, key):
if len(self.cache) >= self.capacity:
self.cache.pop(0)
self.cache.append(key)
```
LRU算法示例代码:
```python
from collections import OrderedDict
class LRU:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
if key in self.cache:
self.cache.move_to_end(key)
return key
else:
return -1
def put(self, key):
if key in self.cache:
self.cache.move_to_end(key)
elif len(self.cache) >= self.capacity:
self.cache.popitem(last=False)
self.cache[key] = None
```
相关推荐
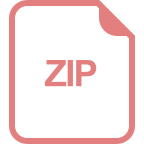
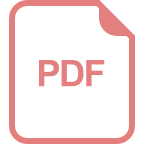















