帮我写一个适用于Dev-c++的顺序表的基本操作实现的代码,并实现顺序表的定义、打印、查找、插入、删除
时间: 2024-09-19 13:04:14 浏览: 42
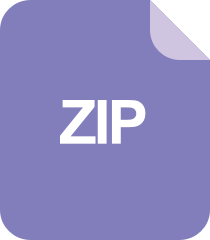
数据结构c++代码(顺序表的代码,包括静态顺序表和动态顺序表)

在Dev-C++中,我们可以创建一个简单的顺序表结构,使用数组作为底层存储,来实现基本的操作。这里是一个基础的顺序表实现示例:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表的节点
struct Node {
int data;
Node* next;
};
// 顺序表类
class LinkedList {
private:
Node* head; // 头指针
int size; // 序列长度
public:
LinkedList() : head(nullptr), size(0) {}
// 插入元素到链表尾部
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
if (head == nullptr) {
head = newNode;
} else {
Node* temp = head;
while (temp->next != nullptr) {
temp = temp->next;
}
temp->next = newNode;
}
size++;
}
// 打印链表
void printList() {
Node* temp = head;
while (temp != nullptr) {
cout << temp->data << " ";
temp = temp->next;
}
cout << endl;
}
// 查找元素
bool find(int key) {
Node* temp = head;
for (int i = 0; i < size && temp != nullptr; i++) {
if (temp->data == key) {
return true;
}
temp = temp->next;
}
return false;
}
// 删除元素(如果存在)
void remove(int key) {
if (head == nullptr || head->data != key) {
return;
}
Node* temp = head;
head = head->next;
delete temp;
size--;
}
};
int main() {
LinkedList list;
list.insert(1);
list.insert(2);
list.insert(3);
cout << "Original List: ";
list.printList();
if (list.find(2)) {
cout << "Found element 2" << endl;
} else {
cout << "Element not found" << endl;
}
list.remove(2);
cout << "After removing 2: ";
list.printList();
return 0;
}
```
阅读全文
相关推荐
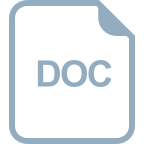
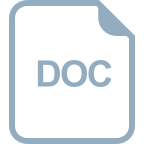
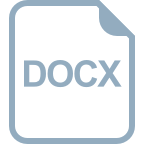
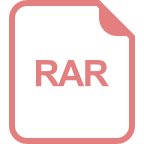
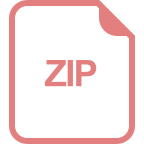
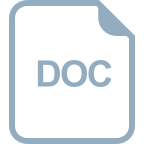
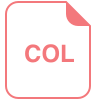










