用c语言建立一个带头节点的单列表。节点的值域为整形数据对该表进行增删改查等各项基本操作,对各项操作的时间复杂度进行分析,写成一个完整的程序
时间: 2024-10-19 16:11:26 浏览: 24
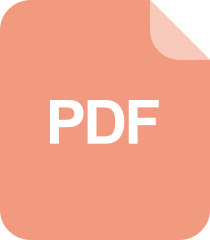
C语言数据结构实现链表逆序并输出
在C语言中,我们可以创建一个结构体来表示链表的节点,并包含整型数据。这里是一个简单的实现,包括头节点的定义、链表的基本操作(插入、删除、查找和修改),以及时间复杂度分析。
首先,我们定义链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
typedef struct Node {
int data; // 节点值
struct Node* next; // 指向下一个节点的指针
} ListNode;
```
接下来,实现链表的操作:
1. 插入节点(`insert`):
- 时间复杂度:O(1) (如果在链表末尾插入),O(n) (在中间位置插入)
```c
void insert(ListNode** head, int value) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
2. 删除节点(`delete`):
- 时间复杂度:O(n) (最坏情况,需遍历整个链表找到目标节点)
```c
void deleteNode(ListNode** head, int value) {
if (*head == NULL) return;
if ((*head)->data == value) {
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
ListNode* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
```
3. 查找节点(`search`):
- 时间复杂度:O(n) (需要遍历整个链表)
```c
ListNode* search(ListNode* head, int value) {
ListNode* current = head;
while (current != NULL) {
if (current->data == value) return current;
current = current->next;
}
return NULL;
}
```
4. 修改节点(`update`):
- 时间复杂度:O(n) (最坏情况下需遍历链表找到目标节点)
```c
void updateNode(ListNode** head, int old_value, int new_value) {
ListNode* current = *head;
while (current != NULL) {
if (current->data == old_value) {
current->data = new_value;
break;
}
current = current->next;
}
}
```
完整程序示例:
```c
int main() {
// 初始化一个空链表
ListNode* head = NULL;
// ... 这里调用上述函数进行操作 ...
return 0;
}
```
阅读全文
相关推荐
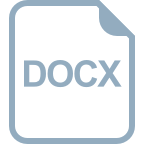
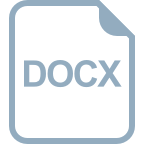


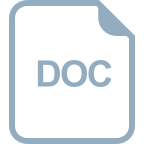
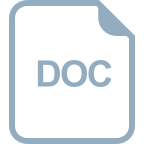
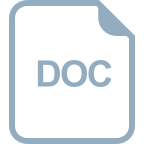

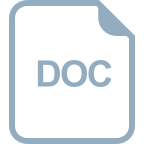
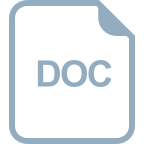
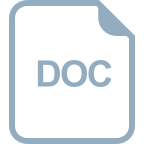
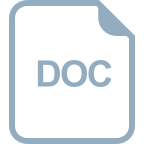
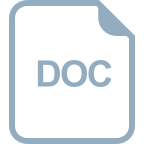
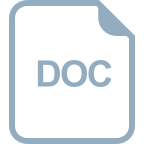
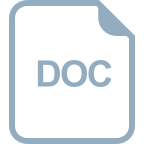

