用c语言进行编写该题
时间: 2024-10-21 20:07:09 浏览: 13
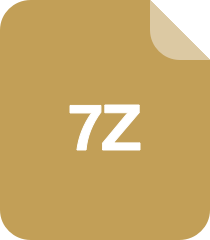
山西专升本C语言历年真题
在C语言中编写这个程序需要考虑到输入、计算和输出的过程。由于C语言的标准库函数`scanf`用于从标准输入读取数据,`printf`用于输出结果,我们可以按照下面的步骤来编写:
```c
#include <stdio.h>
// 假设我们定义一个结构体来存储艺人信息
typedef struct {
char name[10];
int audience_votes;
int judge_votes;
} Artist;
// 函数来获取艺人胜利
Artist get_winner(Artist a, Artist b, int* judge_votes) {
// 初始化艺术家的总票数
a.total_votes = a.audience_votes + judge_votes[0] + judge_votes[1] + judge_votes[2];
b.total_votes = b.audience_votes + judge_votes[0] + judge_votes[1] + judge_votes[2];
if (a.total_votes > b.total_votes || (a.total_votes == b.total_votes && judge_votes[0] > judge_votes[1])) {
return a;
} else {
return b;
}
}
int main() {
// 读取输入
int Pa, Pb;
scanf("%d %d", &Pa, &Pb);
Artist artist_a = {"a", Pa, 0};
Artist artist_b = {"b", Pb, 0};
// 获取评委投票
int judge1, judge2, judge3;
scanf("%d %d %d", &judge1, &judge2, &judge3);
// 将评委投票数组传递给get_winner函数
artist_a.judge_votes = judge1;
artist_b.judge_votes = judge2 + judge3;
Artist winner = get_winner(artist_a, artist_b, &artist_b.judge_votes); // 使用b的评委投票作为整体
// 输出结果
printf("The winner is %s: %d\n", winner.name, winner.total_votes);
return 0;
}
```
在这个代码中,首先定义了一个名为`Artist`的结构体,然后定义了一个`get_winner`函数来计算两位艺人的总票数并确定胜利者。在`main`函数中,读取观众票数和评委票数,调用`get_winner`函数,最后输出胜利者及其票数。
阅读全文
相关推荐
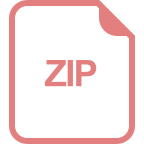
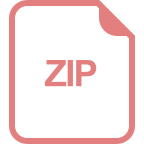
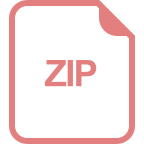
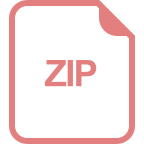
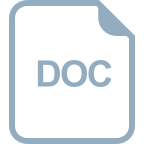
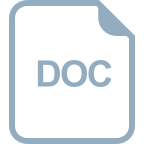
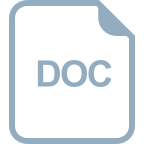
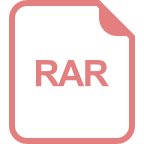
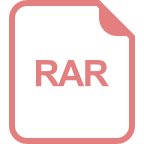
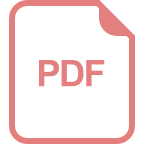
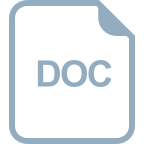

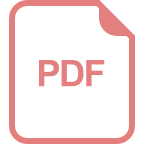
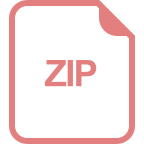
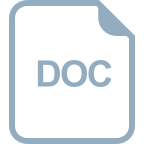
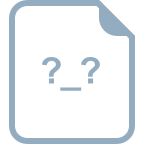