5.编程实现:某航空公司规定在旅游旺季(7~9 月),票价九折优惠;在旅游淡季 (3~5 月、11 月),票价六折优惠;其他月份,票价八折优惠。根据输入的月份及票价,计算并输出应付金额。
时间: 2024-10-11 17:09:34 浏览: 38
在编程中,你可以使用条件语句(如if-else if-else结构)来实现这个需求。这里是一个简单的Python示例:
```python
def calculate_ticket_cost(month, base_price):
if month in [7, 8, 9]: # 旺季
discount = 0.9
elif month in [3, 4, 5] or month == 11: # 淡季
discount = 0.6
else: # 其他月份
discount = 0.8
discounted_price = base_price * discount
return discounted_price
# 使用示例
base_price = float(input("请输入基础票价:"))
month = int(input("请输入月份:"))
result = calculate_ticket_cost(month, base_price)
print(f"应付金额为:{result}元")
```
用户需要输入基础票价和月份,程序会根据规定的折扣规则计算出实际应付金额。
相关问题
5.编程实现:某航空公司规定在旅游旺季(7~9 月),票价九折优惠;在旅游淡季(3~5 月、11 月),票价六折优惠;其他月份,票价八折优惠。根据输入的月份及票价,计算并输出应付金额
在Python中,你可以创建一个函数来处理这种条件折扣。这里是一个简单的例子:
```python
def calculate_ticket_price(month, base_price):
if month in [7, 8, 9]: # 旅游旺季
discount = 0.9
elif month in [3, 4, 5] or month == 11: # 旅游淡季
discount = 0.6
else: # 其他月份
discount = 0.8
discounted_price = base_price * discount
return discounted_price
# 测试函数
base_price = float(input("请输入基础票价:"))
month = int(input("请输入月份(1-12):"))
result = calculate_ticket_price(month, base_price)
print(f"应付金额为:{result}元")
```
这个函数首先检查输入的月份是否属于旺季、淡季或其他情况,然后计算相应的折扣,并将折扣应用到基本票价上。用户可以输入基础票价和月份,程序会返回相应的应付金额。
c语言5.编程实现:某航空公司规定在旅游旺季(7~9 月),票价九折优惠;在旅游淡季(3~5 月、11 月),票价六折优惠;其他月份,票价八折优惠。根据输入的月份及票价,计算并输出应付金额。
在C语言中,你可以使用if...elif...else语句来实现这个折扣计算功能。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h> // 使用strlen获取字符串长度
int main() {
char month[10];
double original_price, discount_rate, total_amount;
printf("请输入月份(格式:mm):");
fgets(month, sizeof(month), stdin); // 获取输入的月份
month[strcspn(month, "\n")] = '\0'; // 移除fgets读取到的换行符
// 根据月份判断折扣率
if (strcmp(month, "07") || strcmp(month, "08") || strcmp(month, "09")) { // 旅游旺季
discount_rate = 0.9; // 九折
} else if (strcmp(month, "03") || strcmp(month, "04") || strcmp(month, "05") || strcmp(month, "11")) { // 旅游淡季
discount_rate = 0.6; // 六折
} else { // 其他月份
discount_rate = 0.8; // 八折
}
printf("请输入原票价:");
scanf("%lf", &original_price);
total_amount = original_price * discount_rate;
printf("应付金额是:%.2f元\n", total_amount);
return 0;
}
```
在这个程序中,首先用户输入月份,然后根据月份字符串判断折扣率,接着获取原价并计算最终应付金额,最后将结果输出。
阅读全文
相关推荐
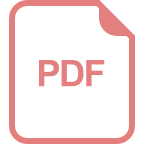
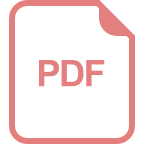
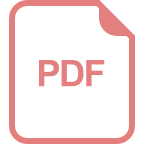













