静态方法不在Spring容器管理的类中,怎么使用构造函数注入或者使用Spring AOP来间接地在运行时动态装配Mapper
时间: 2024-10-01 12:06:21 浏览: 10
如果静态方法不在Spring容器管理的类中,我们可以使用以下两种方式来间接地在运行时动态装配Mapper:
1. **构造函数注入**:
- 创建一个独立的服务类,该类有一个构造函数接受Mapper作为参数:
```java
public class StaticService {
private final YourMapper mapper;
public StaticService(YourMapper mapper) {
this.mapper = mapper;
}
public static void yourStaticMethod(int id) {
StaticService service = new StaticService(getYourMapper()); // 构造时注入Mapper
YourEntity entity = service.getYourEntityById(id);
}
private YourEntity getYourEntityById(int id) {
return mapper.findById(id);
}
}
```
- 在Spring配置文件中,可以创建一个 bean 并指定传入 Mapper 实例:
```xml
<bean id="staticService" class="com.example.StaticService">
<constructor-arg ref="yourMapper"/>
</bean>
```
- 现在静态方法`yourStaticMethod`就可以使用构造函数注入的Mapper了。
2. **Spring AOP (Aspect Oriented Programming)**:
- 定义一个切面(Aspect),使用`@Around`通知来在静态方法调用前注入Mapper:
```java
@Aspect
public class DependencyInjectionAspect {
@Around("@annotation(yourStaticMethod)")
public Object aroundCall(ProceedingJoinPoint joinPoint) throws Throwable {
YourMapper mapper = getYourMapper();
Object result = joinPoint.proceed(new Object[]{mapper}); // 将Mapper作为参数传递给原始方法
return result;
}
private YourMapper getYourMapper() {
return SpringContextUtil.getBean(YourMapper.class);
}
}
```
- 在Spring配置中启用AOP和配置切面:
```xml
<aop:config/>
<bean id="dependencyInjectionAspect" class="com.example.DependencyInjectionAspect"/>
```
- 在静态方法上添加对应的注解,如`@yourStaticMethod`,然后这个方法会在运行时通过AOP动态装配Mapper。
无论是构造函数注入还是AOP,都是为了在不改变静态方法的情况下,让Spring在运行时提供必要的资源。
相关推荐
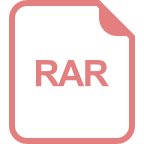
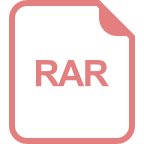
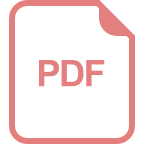














