Java 提供接口实现文件下载 前端生成txt文档
时间: 2023-08-21 22:05:16 浏览: 154
在 Java 中,您可以使用 Spring Boot 框架来提供接口实现文件下载功能。同时,前端可以使用 JavaScript 在浏览器中生成并下载 txt 文档。下面是一个示例代码:
Java 后端代码(使用 Spring Boot):
```java
import org.springframework.core.io.Resource;
import org.springframework.core.io.UrlResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import java.io.IOException;
import java.net.MalformedURLException;
import java.nio.file.Path;
import java.nio.file.Paths;
@Controller
@RequestMapping("/download")
public class DownloadController {
@GetMapping("/txt")
public ResponseEntity<Resource> downloadTxtFile() throws IOException {
// 生成要下载的文本内容
String content = "This is a sample text file.";
// 创建临时文件并写入内容
Path tempFile = Paths.get("temp.txt");
Files.write(tempFile, content.getBytes());
// 创建资源对象
Resource resource = new UrlResource(tempFile.toUri());
// 设置文件下载响应头
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"temp.txt\"")
.contentType(MediaType.TEXT_PLAIN)
.body(resource);
}
}
```
在上述示例代码中,我们创建了一个名为 `DownloadController` 的控制器,其中包含了一个 `/download/txt` 的接口。该接口会生成一个临时的 txt 文件,并返回给前端进行下载。
前端代码(使用 JavaScript):
```html
<!DOCTYPE html>
<html>
<head>
<title>Generate and Download Text File</title>
</head>
<body>
<button onclick="generateAndDownloadFile()">Download Text File</button>
<script>
function generateAndDownloadFile() {
// 生成文本内容
var content = "This is a sample text file.";
// 创建 Blob 对象
var blob = new Blob([content], { type: 'text/plain' });
// 创建下载链接
var downloadLink = document.createElement('a');
downloadLink.href = window.URL.createObjectURL(blob);
downloadLink.download = 'sample.txt';
// 模拟点击下载链接
downloadLink.click();
}
</script>
</body>
</html>
```
在上述示例代码中,我们创建了一个包含一个按钮的 HTML 页面。当用户点击按钮时,会调用 JavaScript 函数 `generateAndDownloadFile()` 来生成并下载 txt 文件。
希望这个示例能够满足您的需求!如果有任何问题,请随时追问。
阅读全文
相关推荐
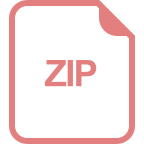
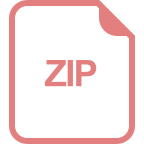
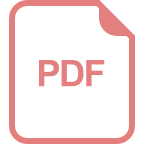
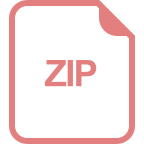
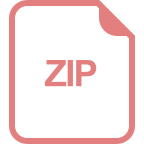
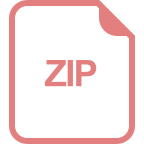
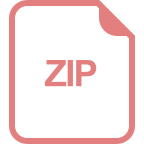
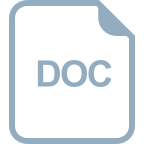
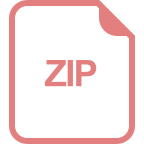
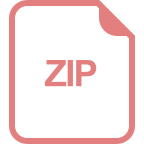
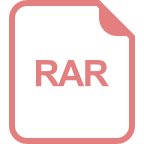
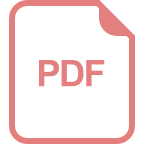
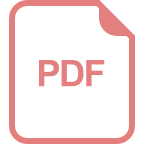
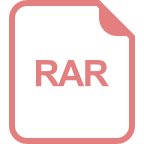
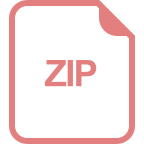
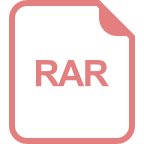