'function' object does not support item assignment
时间: 2024-01-27 07:05:00 浏览: 358
这个错误通常发生在尝试对一个函数对象进行索引赋值时。函数对象是不可改变的,因此不能像列表或字典那样对其进行元素赋值。
例如,对于以下代码:
```python
def my_function():
print("Hello, World!")
my_function[0] = 'H'
```
会抛出 `'function' object does not support item assignment` 错误,因为你不能对 `my_function` 这个函数对象的索引位置进行赋值操作。
如果你想修改函数对象的行为,可以通过定义一个新的函数来实现,或者使用装饰器来包装原始函数。
相关问题
TypeError: 'NoneType' object does not support item assignment
This error occurs when you try to assign a value to an item in an object or variable that has a value of None. None is a special value in Python representing the absence of a value, similar to null in other programming languages.
For example, if you try to assign a value to an item in a function that returns None:
```
def my_function():
# do something
return None
my_function()[0] = 'value'
```
You will get the error "TypeError: 'NoneType' object does not support item assignment" because the function returns None, which is not a mutable object that can be modified by assigning values to its items.
To fix this error, you need to make sure that the object or variable you are trying to modify is not None. You can use an if statement to check if the value is None before trying to modify it:
```
my_return_value = my_function()
if my_return_value is not None:
my_return_value[0] = 'value'
```
Alternatively, you can modify your code to return a mutable object instead of None, such as a list or dictionary, so that you can assign values to its items.
Message='builtin_function_or_method' object does not support item assignment Source=E:\1daer\wanganshijian\6.10\_6.10.py StackTrace: File "E:\1daer\wanganshijian\6.10\_6.10.py", line 4, in <module> (Current frame) input[i] = chr(enc[i] ^ iv) ~~~~~^^^ TypeError: 'builtin_function_or_method' object does not support item assignment
这个错误是因为在 Python 中,函数参数是不可变的,所以不能像 C 语言那样通过下标来修改函数参数的值。解决这个问题的方法是,将函数参数 `input` 定义为一个可以修改的对象,比如列表或者字节数组,然后在函数内部修改该对象的值。
以下是一个修改后的 Python 实现:
```python
def construct_input(input):
enc = [65, 192, 124, 229, 151, 36, 175, 112, 235, 157, 6, 161, 95, 250, 97, 27, 178, 42, 213, 56]
iv = 0x33
for i in range(20):
input[i] = enc[i] ^ iv
iv = input[i] + iv
```
在这个实现中,我们将函数参数 `input` 定义为一个列表,然后在函数内部通过下标来修改列表中相应位置的值。这样就可以避免上述错误的出现。
阅读全文
相关推荐
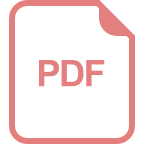
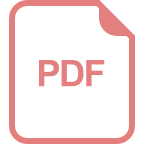
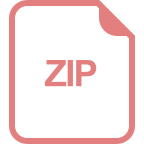


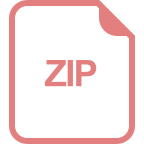
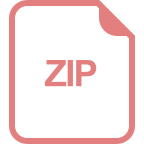
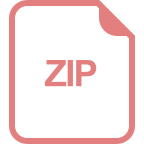