Uncaught EvalError: Identifier 'get_cookie' cannot be declared with 'var' in current evaluation scope, consider trying 'let' instead
时间: 2024-11-05 18:25:30 浏览: 11
这个错误信息通常出现在JavaScript编程中,特别是当你试图在一个eval()函数内声明变量,但该变量名已存在于当前作用域内。`EvalError: Identifier 'get_cookie' cannot be declared with 'var'`表示函数内部的变量`get_cookie`已经在其他地方被声明过了,而在JavaScript的严格模式下,`var`关键字无法在eval环境中重新声明。
在JavaScript中,`var`的作用域是在整个函数,而`let`和`const`(ES6引入的)的作用域则更小,通常是块级作用域,即只在它们所在的代码块里有效。因此,建议你检查一下`get_cookie`是否已在当前eval上下文中声明过,并尝试使用`let`替换`var`来避免冲突:
```javascript
try {
let get_cookie; // 将 var 改为 let,这样就不会覆盖之前的声明
eval("function myFunction() { ... }"); // 确保get_cookie没有在eval之前被使用
} catch (error) {
console.error(error);
}
```
相关问题
libc++abi: terminating with uncaught exception of type std::__1::system_error: condition_variable wait failed: Invalid argument
This error occurs when a condition_variable is waiting for a notification, but the underlying condition is not valid. This can happen if the function is called with an invalid argument or if the condition was not initialized properly.
To fix this error, you need to check the arguments being passed to the condition_variable and ensure that they are valid. You may also need to initialize the condition properly before using it.
Here's an example of how to use a condition_variable:
```
#include <iostream>
#include <condition_variable>
#include <mutex>
#include <thread>
std::condition_variable cv;
std::mutex mtx;
bool ready = false;
void thread_func()
{
std::unique_lock<std::mutex> lock(mtx);
ready = true;
cv.notify_one();
}
int main()
{
std::thread t(thread_func);
std::unique_lock<std::mutex> lock(mtx);
while (!ready) {
cv.wait(lock);
}
t.join();
return 0;
}
```
In this example, we create a condition_variable named `cv`, a mutex named `mtx`, and a boolean flag called `ready`. We start a new thread that sets the `ready` flag to true and notifies the condition_variable. In the main thread, we wait for the notification by calling `cv.wait(lock)` in a loop.
Note that we need to acquire the lock before calling `cv.wait()` or `cv.notify_one()`. This ensures that the condition is modified atomically and prevents race conditions.
If you are still experiencing the error after checking your code, you may need to consult the documentation for the specific function that is throwing the exception to see if there are any known issues or limitations.
libc++abi: terminating with uncaught exception of type std::bad_alloc: std::bad_alloc zsh: abort
这个错误信息表明程序在运行时遇到了std::bad_alloc异常,这通常是由于程序试图分配的内存超出了操作系统或程序所允许的范围。这个异常通常会导致程序崩溃并终止运行。
要解决这个问题,可以尝试以下几种方法:
1. 检查程序中是否有内存泄漏或者过度分配内存的情况,可以使用内存分析工具来帮助检测。
2. 尝试减少程序中对内存的使用,例如使用更高效的算法或数据结构。
3. 增加系统内存或者调整程序的内存限制,以便程序可以分配更多的内存。
阅读全文
相关推荐
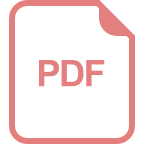
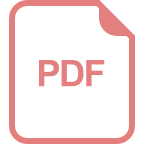
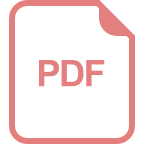













