利用C#拆分Excel工作表
时间: 2023-07-12 17:16:42 浏览: 174
您可以使用 Microsoft.Office.Interop.Excel 库来利用 C# 拆分 Excel 工作表。以下是一个示例代码,演示如何将一个工作表拆分成多个工作表,每个工作表包含指定数量的行:
```csharp
using System;
using System.IO;
using System.Runtime.InteropServices;
using Microsoft.Office.Interop.Excel;
namespace ExcelSplitter
{
class Program
{
static void Main(string[] args)
{
string inputFile = "input.xlsx";
int rowsPerSheet = 100; //每个工作表包含的行数
//启动 Excel 应用程序
Application excel = new Application();
excel.DisplayAlerts = false;
//打开 Excel 文件
Workbook workbook = excel.Workbooks.Open(Path.GetFullPath(inputFile));
//获取原始工作表
Worksheet originalSheet = workbook.Sheets[1];
//计算拆分后的工作表数量
int totalRows = originalSheet.UsedRange.Rows.Count;
int totalSheets = (int)Math.Ceiling((double)totalRows / rowsPerSheet);
//循环创建新工作表
for (int i = 1; i <= totalSheets; i++)
{
//新建工作表
Worksheet newSheet = workbook.Sheets.Add(Type.Missing, originalSheet, Type.Missing, Type.Missing);
newSheet.Name = "Sheet" + i;
//复制指定行数的数据到新工作表
int startRow = (i - 1) * rowsPerSheet + 1;
int endRow = Math.Min(i * rowsPerSheet, totalRows);
Range sourceRange = originalSheet.Range["A" + startRow, "Z" + endRow];
Range destinationRange = newSheet.Range["A1", Type.Missing];
sourceRange.Copy(destinationRange);
}
//保存并关闭 Excel 文件
workbook.Save();
workbook.Close();
excel.Quit();
//释放 COM 对象
Marshal.ReleaseComObject(originalSheet);
Marshal.ReleaseComObject(workbook);
Marshal.ReleaseComObject(excel);
}
}
}
```
请注意,您需要在项目中添加对 Microsoft.Office.Interop.Excel 的引用。此外,该示例代码假定 Excel 文件的第一个工作表包含要拆分的数据。如果您的情况不同,请相应地修改代码。
阅读全文
相关推荐
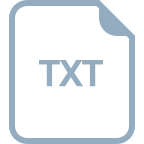
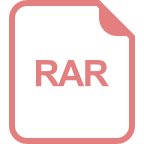
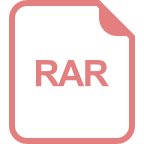










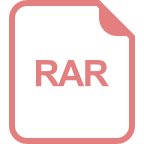
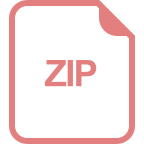
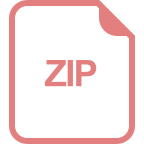
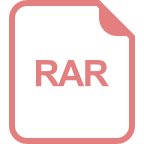
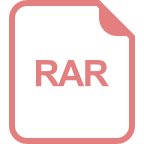
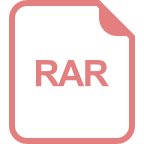