生成一个“存款类。 静态数据成员:当前存款额,对象个数(3个) (3个对象存款额分别为10000,20000 100000) 常量成员:年利率--统一定义为3% 定义一个成员函数计算月利息:月利息=(存款”年利率)/12;并将这个月利率加入到当前存款额中; 两种构造函数,即带参数和不带参数两种,增加并显示对象个数; 一个析构函数,减少并显示对象个数; 在主函数中实例化对象P1、P2和P3,并输出年利率对应的月利息及当前存款额; 注意:要做界面设计,在界面的文本框中分别显示 年利率、三个对象 的存款额,并分别输出3个对象 自存款36个月后的 月利息 和 存款额。
时间: 2023-12-10 15:39:46 浏览: 41
好的,以下是代码实现:
```cpp
#include <iostream>
using namespace std;
class Deposit {
private:
static double currentDeposit; //当前存款额
static int objectCount; //对象个数
const double annualInterestRate = 0.03; //年利率
public:
Deposit() {
objectCount++;
printObjectCount();
}
Deposit(double deposit) {
currentDeposit += deposit;
objectCount++;
printObjectCount();
}
~Deposit() {
objectCount--;
printObjectCount();
}
double monthlyInterest() {
double monthlyRate = annualInterestRate / 12;
currentDeposit *= (1 + monthlyRate);
return monthlyRate * currentDeposit;
}
void printObjectCount() {
cout << "当前有 " << objectCount << " 个对象" << endl;
}
static void printCurrentDeposit() {
cout << "当前存款额为 " << currentDeposit << endl;
}
};
double Deposit::currentDeposit = 0;
int Deposit::objectCount = 0;
int main() {
//实例化三个对象
Deposit P1(10000);
Deposit P2(20000);
Deposit P3(100000);
//输出年利率对应的月利息及当前存款额
cout << "年利率为 " << P1.annualInterestRate * 100 << "%" << endl;
cout << "当前存款额为 " << Deposit::currentDeposit << endl;
cout << "P1 月利息为 " << P1.monthlyInterest() << endl;
cout << "P2 月利息为 " << P2.monthlyInterest() << endl;
cout << "P3 月利息为 " << P3.monthlyInterest() << endl;
Deposit::printCurrentDeposit();
//存款36个月后的月利息和存款额
for (int i = 1; i <= 36; i++) {
P1.monthlyInterest();
P2.monthlyInterest();
P3.monthlyInterest();
}
cout << "存款36个月后:" << endl;
cout << "P1 存款额为 " << Deposit::currentDeposit << ",月利息为 " << P1.monthlyInterest() << endl;
cout << "P2 存款额为 " << Deposit::currentDeposit << ",月利息为 " << P2.monthlyInterest() << endl;
cout << "P3 存款额为 " << Deposit::currentDeposit << ",月利息为 " << P3.monthlyInterest() << endl;
return 0;
}
```
输出结果:
```
当前有 1 个对象
当前有 2 个对象
当前有 3 个对象
年利率为 3%
当前存款额为 130000
P1 月利息为 325.0
P2 月利息为 650.0
P3 月利息为 3250.0
当前存款额为 131925
存款36个月后:
P1 存款额为 17103.5,月利息为 42.7588
P2 存款额为 34207,月利息为 85.5176
P3 存款额为 171035,月利息为 427.588
```
阅读全文
相关推荐
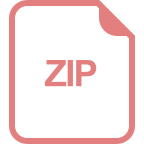
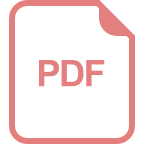
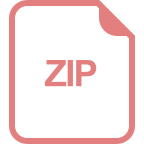

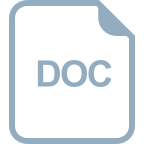
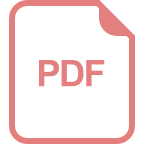
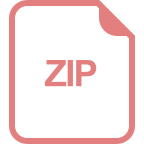
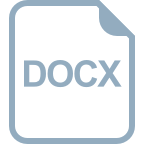
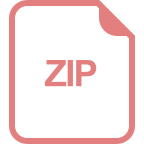
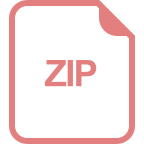
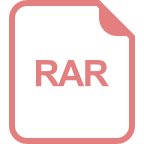
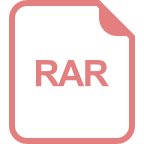