需要关于LCD1602和STM32f103C8T6的连线图
时间: 2023-06-24 18:07:18 浏览: 73
以下是LCD1602和STM32F103C8T6的连线图:

其中,VSS、VDD、VEE分别接地、电源和对比度控制电压,RS、RW、EN分别为指令/数据选择、读写控制、使能控制,D0~D7为数据线,A、K为背光正负极。
具体连线方式如下:
- VSS接GND
- VDD接5V
- VEE通过一个10K电位器接电源负极(GND),调节对比度
- RS接PA1
- RW接GND
- EN接PA0
- D0~D7接PB0~PB7
- A接5V
- K接GND
同时,需要注意的是,在使用STM32F103C8T6驱动LCD1602时,需要使用4位数据线模式,即只使用D4~D7这四根数据线。具体实现方式可以参考以下代码:
```
#define LCD_DATA_GPIO GPIOB
#define LCD_RS_GPIO GPIOA
#define LCD_EN_GPIO GPIOA
#define LCD_D4_PIN GPIO_Pin_0
#define LCD_D5_PIN GPIO_Pin_1
#define LCD_D6_PIN GPIO_Pin_2
#define LCD_D7_PIN GPIO_Pin_3
#define LCD_RS_PIN GPIO_Pin_1
#define LCD_EN_PIN GPIO_Pin_0
#define LCD_DATA_MASK (LCD_D4_PIN | LCD_D5_PIN | LCD_D6_PIN | LCD_D7_PIN)
void LCD_WriteNibble(uint8_t nibble, uint8_t rs) {
if (rs) {
GPIO_SetBits(LCD_RS_GPIO, LCD_RS_PIN);
} else {
GPIO_ResetBits(LCD_RS_GPIO, LCD_RS_PIN);
}
GPIO_SetBits(LCD_EN_GPIO, LCD_EN_PIN);
GPIO_Write(LCD_DATA_GPIO, (GPIO_ReadInputData(LCD_DATA_GPIO) & ~LCD_DATA_MASK) | (nibble << 0));
GPIO_ResetBits(LCD_EN_GPIO, LCD_EN_PIN);
}
void LCD_WriteByte(uint8_t byte, uint8_t rs) {
LCD_WriteNibble(byte >> 4, rs);
LCD_WriteNibble(byte & 0x0F, rs);
}
void LCD_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB, ENABLE);
GPIO_InitStructure.GPIO_Pin = LCD_RS_PIN | LCD_EN_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LCD_RS_GPIO, &GPIO_InitStructure);
GPIO_Init(LCD_EN_GPIO, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = LCD_D4_PIN | LCD_D5_PIN | LCD_D6_PIN | LCD_D7_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LCD_DATA_GPIO, &GPIO_InitStructure);
// 初始化过程
GPIO_ResetBits(LCD_RS_GPIO, LCD_RS_PIN);
GPIO_ResetBits(LCD_EN_GPIO, LCD_EN_PIN);
GPIO_ResetBits(LCD_DATA_GPIO, LCD_DATA_MASK);
Delay_ms(15);
LCD_WriteNibble(0x03, 0);
Delay_ms(5);
LCD_WriteNibble(0x03, 0);
Delay_ms(1);
LCD_WriteNibble(0x03, 0);
Delay_ms(1);
LCD_WriteNibble(0x02, 0);
LCD_WriteByte(0x28, 0);
LCD_WriteByte(0x0C, 0);
LCD_WriteByte(0x06, 0);
LCD_WriteByte(0x01, 0);
}
void LCD_Clear(void) {
LCD_WriteByte(0x01, 0);
Delay_ms(2);
}
void LCD_WriteChar(uint8_t ch) {
LCD_WriteByte(ch, 1);
}
void LCD_WriteString(const char *str) {
while (*str) {
LCD_WriteChar(*str++);
}
}
```
相关推荐
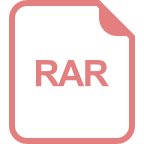
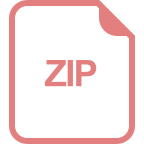













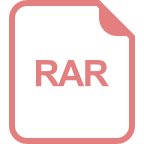