uniapp做微信小程序怎么实现将图片做成地图,能在上面标记打点
时间: 2023-07-19 16:07:47 浏览: 155
要将图片做成地图并在上面标记打点,可以使用以下步骤:
1. 在 `pages` 目录下创建一个新的页面,比如叫做 `map.vue`。
2. 在 `map.vue` 页面中,使用 `image` 组件来显示图片,并设置宽度、高度等样式。例如:
```
<template>
<view>
<image src="../../static/map.jpg" style="width: 100%; height: 100%;"></image>
</view>
</template>
```
3. 在 `map.vue` 页面中,使用 `canvas` 组件来绘制打点。首先需要获取 `canvas` 的上下文,然后使用 `drawImage` 方法将图片绘制到 `canvas` 上。接着,使用 `fillStyle` 和 `fillRect` 方法绘制圆点。
```
<template>
<view>
<image src="../../static/map.jpg" style="width: 100%; height: 100%;"></image>
<canvas id="canvas" style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></canvas>
</view>
</template>
<script>
export default {
onReady() {
const ctx = uni.createCanvasContext('canvas', this);
ctx.drawImage('../../static/map.jpg', 0, 0, uni.getSystemInfoSync().windowWidth, uni.getSystemInfoSync().windowHeight);
ctx.fillStyle = "red";
ctx.fillRect(100, 100, 10, 10);
ctx.draw();
},
};
</script>
```
4. 在 `map.vue` 页面中,可以通过 `touchstart` 事件获取用户的点击位置,并在该位置绘制圆点。
```
<template>
<view>
<image src="../../static/map.jpg" style="width: 100%; height: 100%;"></image>
<canvas id="canvas" style="position: absolute; top: 0; left: 0; width: 100%; height: 100%;"></canvas>
</view>
</template>
<script>
export default {
onReady() {
const ctx = uni.createCanvasContext('canvas', this);
ctx.drawImage('../../static/map.jpg', 0, 0, uni.getSystemInfoSync().windowWidth, uni.getSystemInfoSync().windowHeight);
ctx.draw();
},
methods: {
onTouchStart(e) {
const { x, y } = e.touches[0];
const ctx = uni.createCanvasContext('canvas', this);
ctx.fillStyle = "red";
ctx.beginPath();
ctx.arc(x, y, 5, 0, 2 * Math.PI);
ctx.fill();
ctx.draw(true);
},
},
};
</script>
```
5. 在 `map.vue` 页面中,可以通过 `touchmove` 事件实现拖拽效果。具体实现方式是在 `touchmove` 事件中记录当前手指位置和上一次手指位置的差值,并将 `canvas` 的位置进行相应的偏移。
```
<template>
<view @touchstart="onTouchStart" @touchmove="onTouchMove" @touchend="onTouchEnd">
<image src="../../static/map.jpg" style="width: 100%; height: 100%;"></image>
<canvas id="canvas" style="position: absolute; top: {{canvasTop}}px; left: {{canvasLeft}}px; width: 100%; height: 100%;"></canvas>
</view>
</template>
<script>
export default {
data() {
return {
canvasTop: 0,
canvasLeft: 0,
lastX: 0,
lastY: 0,
};
},
onReady() {
const ctx = uni.createCanvasContext('canvas', this);
ctx.drawImage('../../static/map.jpg', 0, 0, uni.getSystemInfoSync().windowWidth, uni.getSystemInfoSync().windowHeight);
ctx.draw();
},
methods: {
onTouchStart(e) {
this.lastX = e.touches[0].clientX;
this.lastY = e.touches[0].clientY;
},
onTouchMove(e) {
const dx = e.touches[0].clientX - this.lastX;
const dy = e.touches[0].clientY - this.lastY;
this.lastX = e.touches[0].clientX;
this.lastY = e.touches[0].clientY;
this.canvasTop += dy;
this.canvasLeft += dx;
},
},
};
</script>
```
这样,就可以在 `map.vue` 页面中将图片做成地图,并在上面标记打点。
阅读全文
相关推荐
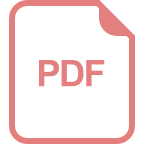

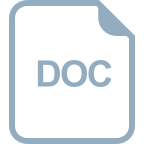



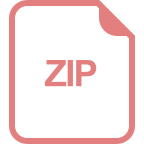
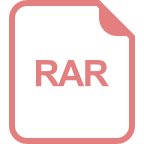
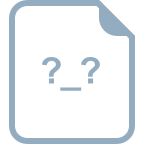
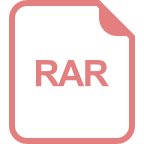
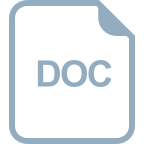


