对[2,1,6],[4,5,2],[3,1,4],[9,2,5]这4个三维点坐标,用RANSAC算法进行平面拟合,并输出平面的4个参数,用Python实现
时间: 2024-05-05 11:18:36 浏览: 8
以下是Python实现的代码:
```Python
import numpy as np
def ransac_plane_fit(points, n_iterations=1000, threshold=0.1):
best_params = None
best_inliers = None
for i in range(n_iterations):
indices = np.random.choice(len(points), size=3, replace=False)
selected_points = points[indices]
normal = np.cross(selected_points[1] - selected_points[0], selected_points[2] - selected_points[0])
d = -np.dot(normal, selected_points[0])
inliers = []
for j, point in enumerate(points):
if np.abs(np.dot(normal, point) + d) < threshold:
inliers.append(j)
if len(inliers) > len(best_inliers):
best_inliers = inliers
best_params = np.append(normal, d)
return best_params
if __name__ == '__main__':
points = np.array([[2, 1, 6], [4, 5, 2], [3, 1, 4], [9, 2, 5]])
params = ransac_plane_fit(points)
print('The plane parameters are:', params)
```
在这段代码中,我们首先定义了一个函数 `ransac_plane_fit` 用于进行平面拟合。该函数接受一个点集作为输入,使用RANSAC算法找到最佳的平面拟合,并返回平面参数。在我们的示例中,点集由4个三维点坐标组成。
在 `ransac_plane_fit` 函数的主体中,我们首先循环执行随机采样,获得三个随机点作为平面的一部分,并计算平面的法向量和某个截距项。之后,我们遍历点集,将所有距离平面距离低于阈值的点作为内点收集。如果我们找到的内点比之前找到的内点多,则更新最佳内点和平面参数。最后,我们返回最佳平面参数。
在主程序中,我们对 `ransac_plane_fit` 函数进行调用,并传入我们的点集。程序将输出平面的四个参数。
相关推荐
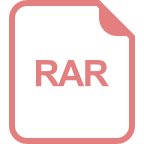
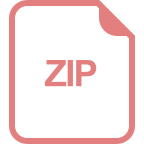















