降序排序算法使用选择法或冒泡法
时间: 2024-05-23 07:10:28 浏览: 15
降序排序可以使用选择排序或冒泡排序,两种算法的思想都比较简单。
选择排序的思想是每次选择未排序部分中的最大值或最小值,将其放到已排序部分的末尾或开头,直到所有元素都排好序。对于降序排序,每次选择未排序部分中的最大值即可。
冒泡排序的思想是每次比较相邻的两个元素,如果它们的顺序不对就交换它们的位置,这样每一轮排序都会让一个元素排好序。对于降序排序,每次比较相邻的两个元素时,如果它们的顺序是升序就交换它们的位置,这样每一轮排序都会让一个较大的元素排好序。
虽然两种算法都可以实现降序排序,但是在时间复杂度上选择排序的最好、最坏和平均时间复杂度都是O(n^2),而冒泡排序的最好时间复杂度是O(n),最坏和平均时间复杂度是O(n^2),因此选择排序的效率略高于冒泡排序。
相关问题
C语言选择排序法和冒泡排序法分别的代码
选择排序法和冒泡排序法都是常用的排序算法,下面是它们的C语言代码实现:
选择排序法:
```c
void selection_sort(int arr[], int n) {
int i, j, min_idx;
// 遍历数组
for (i = 0; i < n-1; i++) {
// 找到未排序部分的最小元素
min_idx = i;
for (j = i+1; j < n; j++) {
if (arr[j] < arr[min_idx]) {
min_idx = j;
}
}
// 将最小元素交换到已排序部分的末尾
int temp = arr[i];
arr[i] = arr[min_idx];
arr[min_idx] = temp;
}
}
```
冒泡排序法:
```c
void bubble_sort(int arr[], int n) {
int i, j;
// 遍历数组
for (i = 0; i < n-1; i++) {
// 每次遍历将最大元素交换到未排序部分的末尾
for (j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
```
以上代码实现的是升序排序。如果需要降序排序,只需要将判断条件改为`arr[j] > arr[min_idx]`和`arr[j] < arr[j+1]`即可。
JAVA 冒泡法排序
### 回答1:
冒泡排序是一种简单的排序算法,它重复地走访过要排序的数列,一次比较两个元素,如果它们的顺序错误就交换它们的位置。具体实现如下:
```java
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// 交换 arr[j] 和 arr[j+1] 的位置
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
```
该算法的时间复杂度为 O(n^2),并且它是一种稳定的排序算法,也就是说,如果两个元素相等,它们在排序后的相对位置不会发生改变。
### 回答2:
冒泡排序是一种基础的排序算法,它的核心思想是通过比较相邻元素的大小,将较大的元素逐渐移到右侧。
具体实现冒泡排序的步骤如下:
1. 首先,遍历待排序的数组,从第一个元素开始,比较相邻的两个元素的大小。
2. 如果当前元素大于下一个元素,则交换它们的位置,将较大的元素向后移动。
3. 继续比较下一个相邻元素,重复上述步骤,直到遍历到数组的最后一个元素。
4. 重复上述步骤,每一轮遍历都会将目前尚未排序的最大元素交换到正确的位置上。
5. 遍历的轮数为数组长度减一,即可完成整个数组的排序。
冒泡排序的时间复杂度为O(n^2),其中n是待排序数组的长度。由于冒泡排序是一种稳定的排序算法,相等元素的相对位置不会发生改变。
要注意的是,冒泡排序算法相对简单但效率较低,特别是在处理大规模数据时,因此不适合使用在大规模数据的排序中。
### 回答3:
冒泡排序是一种简单的排序算法,其基本思想是通过不断比较相邻元素的大小,将较大(或较小)的元素逐步向末尾移动,从而实现排序的目的。
具体实现冒泡排序的步骤如下:
1. 首先,将待排序的元素按照顺序放置在一个数组中。
2. 然后,从数组的第一个元素开始,依次与其相邻的元素进行比较。
3. 如果当前元素比下一个元素大(或小),则交换它们的位置。
4. 继续上述比较和交换的过程,直到将整个数组排序完成。
5. 最后,得到的数组就是一个按照升序(或降序)排列的数组。
冒泡排序的时间复杂度为O(n^2),其中n是待排序元素的个数。空间复杂度为O(1),即不需要额外的空间来存储数组。
这个算法的原理和实现比较简单,但是其效率并不高,因此不适用于大规模数据的排序。不过,在一些特殊情况下,冒泡排序可能会有一些优化的方法,例如设置一个标志位,当某一趟比较没有发生交换时,认为已经有序,从而提前结束排序过程。
相关推荐
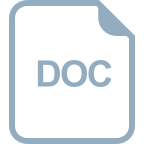
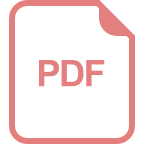
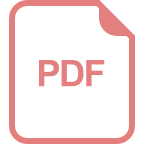












