fastapi启动后台函数
时间: 2023-06-25 10:05:34 浏览: 159
在 FastAPI 中启动后台函数可以使用 Python 的 `asyncio` 模块。下面是一个示例代码:
```python
import asyncio
from fastapi import FastAPI
app = FastAPI()
async def my_background_task():
while True:
await asyncio.sleep(5)
print("Background task is running")
@app.on_event("startup")
async def startup_event():
asyncio.create_task(my_background_task())
```
在这个示例中,我们定义了一个名为 `my_background_task` 的后台任务函数,它将每隔 5 秒钟打印一条消息。然后,我们使用 FastAPI 的 `on_event` 装饰器来注册一个名为 `startup_event` 的事件处理函数。当应用程序启动时,`startup_event` 函数将创建一个 `my_background_task` 任务并将其添加到异步事件循环中。
这样,当 FastAPI 应用程序启动时,`my_background_task` 函数将会在后台运行,并且每隔 5 秒钟打印一条消息。
相关问题
fastapi启动后台函数,但该函数执行中不能再次启动,直到函数运行完成才能再次使用
你可以使用 Python 的 `asyncio` 模块创建一个异步函数来实现这个需求。具体代码如下:
```python
import asyncio
from fastapi import FastAPI
app = FastAPI()
async def background_task():
while True:
# 执行一些后台任务
print("Background task running...")
await asyncio.sleep(10)
@app.on_event("startup")
async def startup_event():
asyncio.create_task(background_task())
@app.post("/some-endpoint")
async def some_endpoint():
# 执行一些操作
print("Some endpoint called.")
```
在这个例子中,我们创建了一个名为 `background_task` 的异步函数,用于执行一些后台任务。在应用启动时,我们调用 `asyncio.create_task` 函数创建一个新的任务,并将 `background_task` 函数作为参数传递给它,从而在后台运行该函数。在 `some_endpoint` 函数中,我们处理客户端的请求,并执行一些操作,但是这个函数不会阻塞 `background_task` 的执行。
请注意,在这个例子中,`background_task` 函数将一直运行,直到应用被关闭,因为我们使用了一个无限循环。如果你想在某个条件成立后停止 `background_task` 的执行,你可以在函数中添加对应的逻辑。
python fastapi,将一个SQLITE数据的表单内容全部显示在html网页上,并可以编辑表格内容,删除数据行,增加数据行,点击保存,保存这些数据到数据库,含CSS和html
FastAPI是一个用于构建Web API的高性能Python框架,它结合了现代Web开发的最佳实践。为了实现你的需求,你需要组合使用FastAPI、Uvicorn(作为HTTP服务器)、HTML/CSS(前端展示)以及一些轻量级库如 SQLAlchemy(操作SQLite数据库)和 Jinja2(模板引擎)。
以下是简化的步骤:
1. **安装依赖**:
- `pip install fastapi uvicorn sqlalchemy jinja2`
- 可能还需要其他CSS框架如`django-crispy-forms`帮助处理表单样式
2. **设置FastAPI应用**:
```python
from fastapi import FastAPI, Depends, HTTPException
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
# 创建数据库连接
engine = create_engine('sqlite:///example.db')
SessionLocal = sessionmaker(autocommit=False, autoflush=False, bind=engine)
Base = declarative_base()
```
3. **创建数据库模型** (例如 UserTable):
```python
class UserTable(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
email = Column(String)
def __repr__(self):
return f'<User {self.name}>'
```
4. **编写CRUD操作函数**:
- 获取所有用户:
```python
async def get_users():
with SessionLocal() as session:
users = session.query(UserTable).all()
return users
```
- 添加/编辑/删除数据:
```python
async def add_user(user: UserTable, db: Session = Depends(SessionLocal)):
db.add(user)
await db.commit()
await db.refresh(user)
return user
# ... 编辑和删除类似实现
```
5. **使用Jinja2模板渲染HTML页面**:
```python
@app.get("/users")
async def show_users(html_view: bool = False):
users = await get_users()
context = {"users": users, "edit_mode": html_view}
return templates.TemplateResponse("index.html", context)
```
6. **index.html** (基本模板结构):
```html
<!-- 使用Jinja2语法嵌入CSS和表单 -->
<style src="styles.css"></style>
<table>
{% for user in users %}
<tr>
<td>{{ user.name }}</td>
<td><input type="text" value="{{ user.email }}" /></td>
<!-- 添加按钮以执行CRUD操作 -->
<td>
<button onclick="deleteRow('{{ user.id }}')">Delete</button>
<button onclick="editRow('{{ user.id }}')">Edit</button>
<button onclick="saveRow('{{ user.id }}')">Save</button>
</td>
</tr>
{% endfor %}
</table>
<!-- 使用JavaScript编写事件处理器,如AJAX请求来与后台交互 -->
<script src="scripts.js"></script>
```
7. **添加CSS和脚本文件**:
- styles.css: 样式规则
- scripts.js: AJAX请求、验证和数据保存逻辑
记得根据实际项目需要调整并完善代码,这只是一个基础示例。运行 FastAPI 应用时,你可以使用 `uvicorn app:app --reload` 启动服务器。
阅读全文
相关推荐
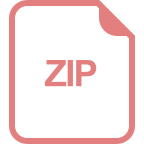
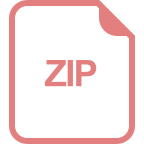
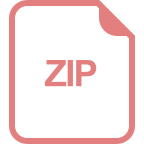
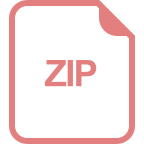
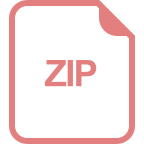
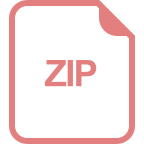
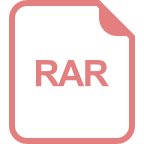
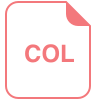
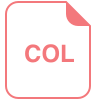
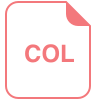
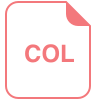
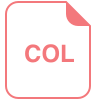
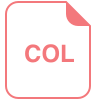
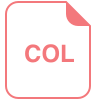
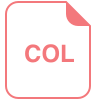
