Python如何在word文档中编辑表格
时间: 2023-11-19 17:12:25 浏览: 121
Python可以使用第三方库`python-docx`来编辑word文档中的表格。
以下是一个简单的示例代码,可以创建一个包含三行两列的表格:
```python
from docx import Document
from docx.shared import Inches
document = Document()
# 创建一个三行两列的表格
table = document.add_table(rows=3, cols=2)
# 设置表格样式
table.style = 'Table Grid'
# 填充表格
table.cell(0, 0).text = '第一行第一列'
table.cell(0, 1).text = '第一行第二列'
table.cell(1, 0).text = '第二行第一列'
table.cell(1, 1).text = '第二行第二列'
table.cell(2, 0).text = '第三行第一列'
table.cell(2, 1).text = '第三行第二列'
# 保存文档
document.save('example.docx')
```
你可以根据自己的需求修改代码,如增加表格行列、设置单元格样式等。
相关问题
python 如何在Word中插入表格
在Python中,通过`python-docx`库可以方便地插入和编辑Word文档中的表格。下面是如何插入一个基本表格的步骤[^1]:
```python
from docx import Document
from docx.table import Table, Cell
# 创建一个新的Word文档
doc = Document()
# 插入一个新表格
table = doc.add_table(rows=3, cols=2) # 3行2列的表格
# 设置表格的第一行标题
header_cells = table.rows[0].cells
header_cells[0].text = "Column 1"
header_cells[1].text = "Column 2"
# 设置表格的其他单元格
for row in table.rows[1:]:
row.cells[0].text = "Row data 1"
row.cells[1].text = "Row data 2"
# 保存文档
doc.save('example.docx')
```
这段代码首先导入了必要的模块,然后创建了一个新的Word文档并添加了一个3行2列的表格。你可以根据需要调整行数、列数以及单元格的内容。
python精确找到并编辑word文档中的表格
在Python中,可以使用`python-docx`库来精确地查找并编辑Word文档中的表格。`python-docx`是一个用于读取、修改和创建Microsoft Word .docx文件的强大工具。以下是基本步骤:
1. 首先,安装`python-docx`库,如果尚未安装,可以使用pip命令安装:
```
pip install python-docx
```
2. 导入必要的模块:
```python
from docx import Document
from docx.table import Table
```
3. 打开Word文档:
```python
document = Document('your_word_file.docx')
```
4. 查找特定表格。你可以通过索引来定位到表格,比如通过标题行找到表格:
```python
for table in document.tables:
if '标题行' in table.cell(0, 0).text: # 这里假设第一列第一行是标题行
target_table = table
break
```
5. 编辑表格内容。例如,替换某一行或单元格的内容:
```python
row_index = 3 # 要操作的行
cell_index = 1 # 要操作的单元格
target_cell = target_table.cell(row_index, cell_index)
target_cell.text = '新内容'
```
6. 最后,保存修改后的文档:
```python
document.save('modified_word_file.docx')
```
阅读全文
相关推荐
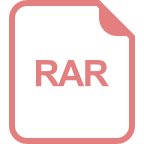
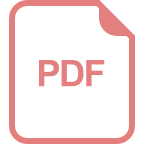
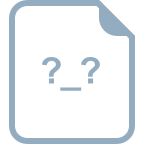













