java如何跨域下载另外一台电脑上面的文件
时间: 2024-09-13 10:03:45 浏览: 55
在Java中实现跨域下载文件通常涉及到网络编程,可以通过多种方式来完成,比如使用HTTP协议来获取远程服务器上的文件。以下是一个简单的例子,说明如何使用Java的`HttpURLConnection`类来下载跨域的文件:
```java
import java.io.BufferedInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class DownloadFile {
public static void downloadFile(String fileURL, String savePath) {
try {
// 创建URL对象
URL url = new URL(fileURL);
// 打开连接
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 允许输入输出
connection.setDoInput(true);
connection.setDoOutput(false);
// 设置请求属性,比如用户代理、接受类型等
connection.setRequestProperty("User-Agent", "Mozilla/5.0");
connection.setRequestProperty("Accept", "image/png,*/*");
// 获取输入流
InputStream inputStream = new BufferedInputStream(connection.getInputStream());
// 输出文件
FileOutputStream outputStream = new FileOutputStream(savePath);
// 用于存储下载文件的字节数组缓冲区
byte[] buffer = new byte[4096];
int bytesRead;
// 读取数据并写入文件
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
// 关闭输入输出流
outputStream.close();
inputStream.close();
connection.disconnect();
System.out.println("下载完成");
} catch (Exception e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
String fileURL = "http://example.com/somefile.txt"; // 远程文件的URL
String savePath = "C:/downloadedfile.txt"; // 本地保存路径
downloadFile(fileURL, savePath);
}
}
```
这段代码展示了如何使用Java的标准库来下载一个文件。首先,它创建了一个`URL`对象,然后通过该URL打开一个`HttpURLConnection`。接下来,通过设置必要的请求属性,并打开输入流,从远程服务器读取文件内容。最后,将读取的内容写入本地文件系统。
在实际应用中,可能需要处理更多的细节,例如认证(基本认证或摘要认证)、错误处理、HTTPS连接等。
阅读全文
相关推荐
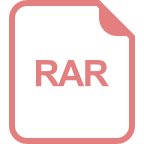
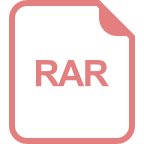
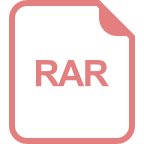
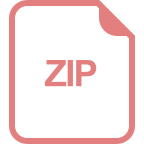
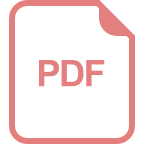
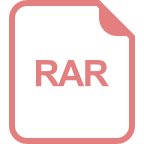
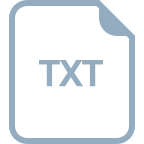
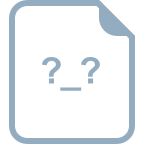
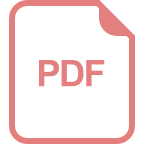
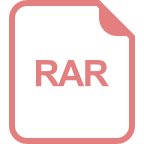
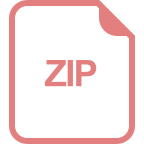
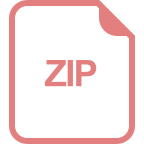
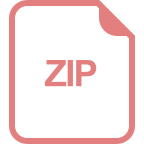
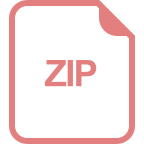
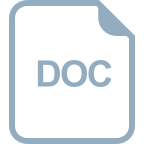