c++遍历多个excel,然后遍历excel中多个sheet
时间: 2023-10-29 19:04:43 浏览: 321
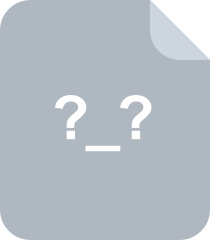
读取Excel文件中多个sheet
你可以使用C++的第三方库来读取和操作Excel文件。常用的有libxl、ExcelFormat和OpenXLSX。这里以libxl为例,介绍如何遍历多个Excel文件和多个工作表。
首先,你需要在你的C++项目中添加libxl库,并包含头文件:
```c++
#include "libxl.h"
using namespace libxl;
```
然后,遍历多个Excel文件,可以使用以下代码:
```c++
int main()
{
Book* book = nullptr;
std::vector<std::string> excelFiles = {"file1.xlsx", "file2.xlsx"}; // 多个Excel文件
for (const auto& excelFile : excelFiles) {
book = xlCreateXMLBook();
if (book->load(excelFile.c_str())) {
int sheetCount = book->sheetCount();
for (int i = 0; i < sheetCount; i++) {
Sheet* sheet = book->getSheet(i);
// 处理当前工作表
// ...
}
}
book->release();
}
return 0;
}
```
遍历Excel文件中的多个工作表,可以在遍历Excel文件的循环中再嵌套一个循环:
```c++
int main()
{
Book* book = nullptr;
std::vector<std::string> excelFiles = {"file.xlsx"};
for (const auto& excelFile : excelFiles) {
book = xlCreateXMLBook();
if (book->load(excelFile.c_str())) {
int sheetCount = book->sheetCount();
for (int i = 0; i < sheetCount; i++) {
Sheet* sheet = book->getSheet(i);
int rowCount = sheet->lastRow();
int columnCount = sheet->lastCol();
for (int row = 0; row < rowCount; row++) {
for (int col = 0; col < columnCount; col++) {
const char* cellValue = sheet->readStr(row, col);
// 处理当前单元格
// ...
}
}
}
}
book->release();
}
return 0;
}
```
以上代码可以读取Excel中的所有单元格,你可以根据需要修改代码,只读取某些单元格。
阅读全文
相关推荐

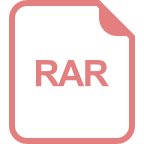
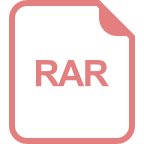
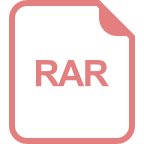
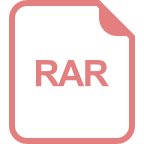
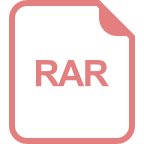
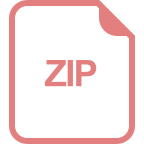
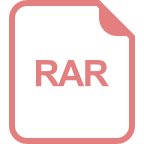
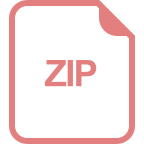
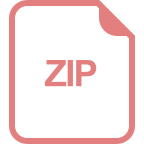
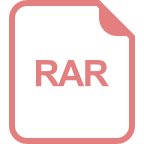
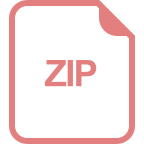



