vue 字体颜色 加粗
时间: 2023-11-07 18:03:41 浏览: 55
在Vue中,可以使用v-bind:style指令来设置字体的样式,包括颜色和加粗。对于字体颜色,你可以在v-bind:style中设置color属性为你想要的颜色值,例如:"red"表示红色。对于加粗,可以使用font-weight属性来设置,可以使用数字值来表示不同的字体粗细,例如700表示粗体。所以,如果你想要在Vue中设置字体颜色为红色并加粗,可以这样写:
<div v-bind:style="{ color: 'red', fontWeight: 700 }"></div>
相关问题
vue-quill-editor加粗失效
如果你在使用 `vue-quill-editor` 编辑器时,发现加粗等功能失效,可能是因为缺少了 Quill 的样式文件。
解决方法是在你的 `index.html` 文件头部加入以下代码来引入 Quill 的样式文件:
```html
<link href="//cdn.quilljs.com/1.3.6/quill.snow.css" rel="stylesheet">
```
如果你已经正确引入了 Quill 的样式文件,但是加粗等功能仍然失效,可能是因为你的配置项不正确。可以参考以下代码进行配置:
```javascript
import { quillEditor } from 'vue-quill-editor'
export default {
components: {
quillEditor
},
data() {
return {
editorOption: {
modules: {
toolbar: [
['bold', 'italic', 'underline', 'strike'], // 加粗,斜体,下划线,删除线
['blockquote', 'code-block'], // 引用,代码块
[{ 'header': 1 }, { 'header': 2 }], // 标题,键值对的形式;1、2表示字体大小
[{ 'list': 'ordered' }, { 'list': 'bullet' }], // 列表
[{ 'script': 'sub' }, { 'script': 'super' }], // 上下标
[{ 'indent': '-1' }, { 'indent': '+1' }], // 缩进
[{ 'direction': 'rtl' }], // 文本方向
[{ 'size': ['small', false, 'large', 'huge'] }], // 字体大小
[{ 'header': [1, 2, 3, 4, 5, 6, false] }], // 几级标题
[{ 'color': [] }, { 'background': [] }], // 字体颜色,字体背景颜色
[{ 'font': [] }], // 字体
[{ 'align': [] }], // 对齐方式
['clean'], // 清除文本格式
['link', 'image', 'video'] // 链接、图片、视频
]
},
// 其他配置项
},
content: ''
}
}
}
```
其中,`toolbar` 数组中包含了所有可用的工具栏功能,包括加粗、斜体、下划线、删除线等。确保你的配置项中包含了这些功能即可正常使用加粗等功能。
vue3 xlsx如何将表头加粗
在vue3 xlsx中,可以通过设置样式来实现表头加粗。具体步骤如下:
1. 导入样式相关的库
```javascript
import XLSX from 'xlsx';
import { write } from 'xlsx-style';
```
2. 创建样式
```javascript
const headerStyle = {
font: {
bold: true, // 加粗
color: { rgb: 'FFFFFF' }, // 字体颜色
},
fill: {
bgColor: { rgb: '2F75B5' }, // 背景颜色
},
};
```
3. 将样式应用到表头
假设表头数据保存在headers数组中,将样式应用到表头的方法如下:
```javascript
const sheetHeaders = headers.map((header) => {
return {
v: header,
s: headerStyle,
};
});
```
4. 将表头数据写入Excel文件
```javascript
const worksheet = XLSX.utils.json_to_sheet(sheetData);
worksheet['!cols'] = [{ width: 15 }, { width: 20 }, { width: 25 }]; // 设置列宽
worksheet['!rows'] = [{ hpx: 30 }]; // 设置行高
worksheet['!merges'] = [{ s: { r: 0, c: 0 }, e: { r: 0, c: 2 } }]; // 合并单元格
worksheet['A1'].s = headerStyle; // 设置A1单元格样式
const workbook = XLSX.utils.book_new();
XLSX.utils.book_append_sheet(workbook, worksheet, 'Sheet1');
write(workbook, { bookType: 'xlsx', type: 'buffer' });
```
通过以上步骤,可以将表头加粗并应用到Excel文件中。
相关推荐
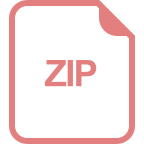












