java生成临时文件不写磁盘 写内存
时间: 2023-09-06 20:02:50 浏览: 246
在Java中生成临时文件并不一定要将其写入硬盘,可以选择将其写入内存中。
通常情况下,我们可以使用`java.nio.file.Files`类提供的`createTempFile`方法来生成临时文件。该方法会在默认的临时文件目录中创建一个新的临时文件,并返回其路径。
如果希望将临时文件写入内存,可以使用Java的内存映射文件(MappedByteBuffer)来实现。内存映射文件是一种将磁盘文件直接映射到内存的技术,可以在不进行文件I/O的情况下访问文件数据。
首先,我们可以使用`java.nio.file.Files`类提供的`createTempFile`方法来创建一个临时文件。然后,我们可以使用`java.nio.channels.FileChannel`类的`map`方法将该临时文件映射到内存中的`MappedByteBuffer`对象。
以下是一个简单的示例代码:
```java
import java.io.IOException;
import java.nio.ByteBuffer;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
public class TempFileInMemoryExample {
public static void main(String[] args) {
try {
Path tempFilePath = Files.createTempFile("temp", ".txt");
// 将临时文件映射到内存中
FileChannel fileChannel = FileChannel.open(tempFilePath, StandardOpenOption.READ, StandardOpenOption.WRITE);
MappedByteBuffer mappedByteBuffer = fileChannel.map(FileChannel.MapMode.READ_WRITE, 0, fileChannel.size());
// 向内存中的映射文件写入数据
String data = "Hello, World!";
byte[] bytes = data.getBytes();
mappedByteBuffer.put(bytes);
// 读取内存中的映射文件数据
mappedByteBuffer.flip();
byte[] readBytes = new byte[bytes.length];
mappedByteBuffer.get(readBytes);
String readData = new String(readBytes);
System.out.println(readData);
// 关闭文件通道
fileChannel.close();
// 删除临时文件
Files.delete(tempFilePath);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在上述代码中,首先创建了一个临时文件,然后将其映射到内存中的`MappedByteBuffer`对象。接着,我们可以向映射文件写入数据,并从中读取数据。最后,关闭文件通道并删除临时文件。
需要注意的是,映射文件将会占用Java虚拟机的堆内存空间,因此在处理大文件时应谨慎使用,避免内存溢出的情况发生。
阅读全文
相关推荐






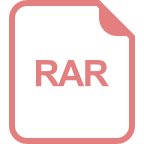










