private string GetNoNumberPart(string numPart, int flag) { int noNumberPart = JZ.Tools.StringHelper.ConvertToInt(numPart) + flag; int totalLength = numPart.Length; string finalNumPart = noNumberPart.ToString(); for (int i = 0; i < totalLength - noNumberPart.ToString().Length; i++) { finalNumPart = "0" + finalNumPart; } return finalNumPart; } private ObjectModel SelectParkingSpace(string no) { ObjectModel obj = ObjectHelper.GetObjectModel(parkingSpace_clsName); string where = $"{obj.GetAttr("BH").ColumnName}='{no}'"; _baseBLL.Select(obj, where); return obj; } public void SetSFFLValue(ObjectModel obj) { ObjectModel objClassify = SelectFeeClassify(obj.GetAttrValue("SYZT"), obj.GetAttrValue("GGCC")); if (!string.IsNullOrEmpty(objClassify.ObjectID)) { obj.SetAttrValue("SFFL", objClassify.GetAttrValue("NAME")); obj.GetAttr("SFFL").RelateSelect.EscapeAttrValue = objClassify.ObjectID; } else { obj.SetAttrValue("SFFL", ""); obj.GetAttr("SFFL").RelateSelect.EscapeAttrValue = ""; } }帮我注释一下上面的代码,谢谢
时间: 2024-02-14 22:07:35 浏览: 67
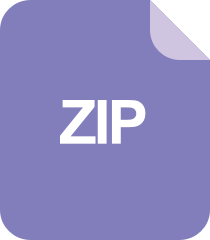
使用CLR集成SQL Alpha数值排序
Sure, here is the commented version of the code:
```
// This method takes a string 'numPart' and an integer 'flag' as input parameters
// It converts the string 'numPart' to an integer, adds the 'flag' to it, and then converts it back to a string
// It then adds leading zeros to the string so that its total length becomes the same as the length of the original 'numPart' string
// Finally, it returns the resulting string
private string GetNoNumberPart(string numPart, int flag)
{
int noNumberPart = JZ.Tools.StringHelper.ConvertToInt(numPart) + flag;
int totalLength = numPart.Length;
string finalNumPart = noNumberPart.ToString();
for (int i = 0; i < totalLength - noNumberPart.ToString().Length; i++)
{
finalNumPart = "0" + finalNumPart;
}
return finalNumPart;
}
// This method takes a string 'no' as input parameter
// It creates an ObjectModel instance using the class name stored in the 'parkingSpace_clsName' variable
// It constructs a string 'where' by concatenating the column name 'BH', the equal sign '=', and the value of 'no' surrounded by single quotes
// It then calls the 'Select' method of the '_baseBLL' object, passing in the ObjectModel instance and the 'where' string as parameters, to select a record from the database
// Finally, it returns the ObjectModel instance with the selected record
private ObjectModel SelectParkingSpace(string no)
{
ObjectModel obj = ObjectHelper.GetObjectModel(parkingSpace_clsName);
string where = $"{obj.GetAttr("BH").ColumnName}='{no}'";
_baseBLL.Select(obj, where);
return obj;
}
// This method takes an ObjectModel instance 'obj' as input parameter
// It calls the 'SelectFeeClassify' method to get an ObjectModel instance 'objClassify' that represents the fee classification of the parking space
// If 'objClassify' is not null, it sets the value of the 'SFFL' attribute of 'obj' to the value of the 'NAME' attribute of 'objClassify'
// It also sets the 'EscapeAttrValue' property of the 'SFFL' attribute to the object ID of 'objClassify'
// If 'objClassify' is null, it sets the value of the 'SFFL' attribute of 'obj' to an empty string
// It also sets the 'EscapeAttrValue' property of the 'SFFL' attribute to an empty string
public void SetSFFLValue(ObjectModel obj)
{
ObjectModel objClassify = SelectFeeClassify(obj.GetAttrValue("SYZT"), obj.GetAttrValue("GGCC"));
if (!string.IsNullOrEmpty(objClassify.ObjectID))
{
obj.SetAttrValue("SFFL", objClassify.GetAttrValue("NAME"));
obj.GetAttr("SFFL").RelateSelect.EscapeAttrValue = objClassify.ObjectID;
}
else
{
obj.SetAttrValue("SFFL", "");
obj.GetAttr("SFFL").RelateSelect.EscapeAttrValue = "";
}
}
```
I hope this helps!
阅读全文
相关推荐
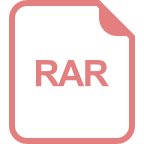
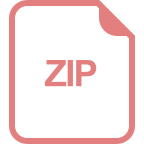

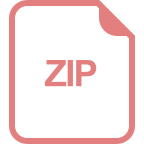
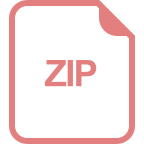
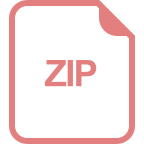
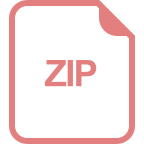
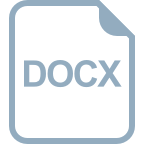
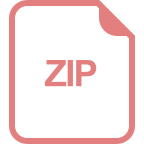
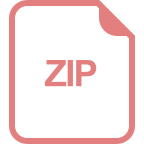
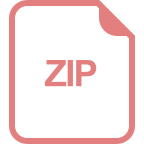
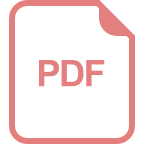
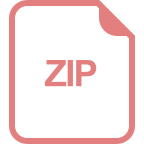
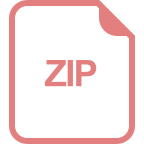
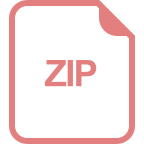
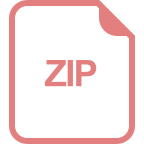
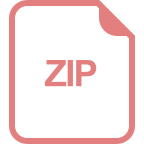