python中替换某个值
时间: 2024-03-21 08:37:37 浏览: 56
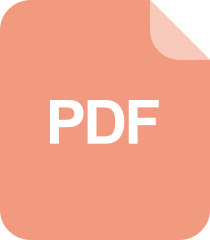
python实现数据清洗(缺失值与异常值处理)

你可以使用Python的字符串方法replace()来替换某个字符串中的某个值,示例代码如下:
```
string = "hello world"
new_string = string.replace("world", "python")
print(new_string) # 输出:hello python
```
在这个例子中,我们将字符串`string`中的"world"替换为"python",并将新字符串赋值给`new_string`。
阅读全文
相关推荐
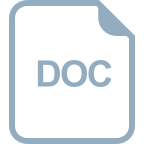
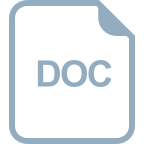

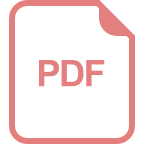
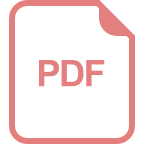












