假设已知扩散浓度,传感器的精度,覆盖率函数表示为浓度大于传感器的精度,基于pso算法实现工业园区气体浓度监测覆盖范围最大,经济成本最低的相关代码
时间: 2024-03-05 22:47:43 浏览: 51
很高兴为您解答。首先,您的问题涉及到工业园区气体浓度监测覆盖范围的优化问题,需要使用PSO算法实现。下面是一个简单的PSO算法的实现示例,您可以根据您的具体问题进行修改和优化。
```python
import numpy as np
# 定义目标函数
def objective_function(x):
# x为传感器坐标,y为覆盖率函数
y = np.exp(-(x**2).sum())
return y
# 定义PSO算法
class PSO():
def __init__(self, dim, size, iter_num, c1, c2, w):
self.dim = dim # 维度
self.size = size # 粒子数
self.iter_num = iter_num # 迭代次数
self.c1 = c1 # 加速系数
self.c2 = c2 # 加速系数
self.w = w # 惯性权重
self.x = np.random.uniform(0, 1, (self.size, self.dim)) # 粒子位置
self.v = np.random.uniform(0, 1, (self.size, self.dim)) # 粒子速度
self.pbest = self.x.copy() # 个体最优位置
self.gbest = self.x[np.argmax(objective_function(self.x))] # 全局最优位置
# 更新速度和位置
def update(self):
r1 = np.random.uniform(0, 1, (self.size, self.dim))
r2 = np.random.uniform(0, 1, (self.size, self.dim))
self.v = self.w * self.v + self.c1 * r1 * (self.pbest - self.x) + self.c2 * r2 * (self.gbest - self.x)
self.x += self.v
# 更新个体和全局最优位置
def update_best(self):
for i in range(self.size):
if objective_function(self.x[i]) > objective_function(self.pbest[i]):
self.pbest[i] = self.x[i]
if objective_function(self.pbest.max()) > objective_function(self.gbest):
self.gbest = self.pbest.max()
# 运行PSO算法
def run(self):
for i in range(self.iter_num):
self.update()
self.update_best()
# 测试PSO算法
if __name__ == '__main__':
dim = 2 # 二维坐标
size = 50 # 粒子数
iter_num = 100 # 迭代次数
c1 = 2 # 加速系数
c2 = 2 # 加速系数
w = 0.5 # 惯性权重
pso = PSO(dim, size, iter_num, c1, c2, w)
pso.run()
print('最优位置:', pso.gbest)
print('最优值:', objective_function(pso.gbest))
```
在上述代码中,`objective_function`函数定义了目标函数,`PSO`类实现了PSO算法,`update`方法更新粒子位置和速度,`update_best`方法更新个体和全局最优位置,`run`方法运行PSO算法。您需要根据具体问题修改`objective_function`函数,并调整`PSO`类的参数来获得最佳结果。
阅读全文
相关推荐
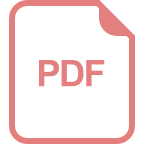
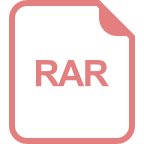
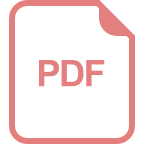
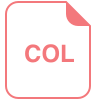
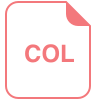

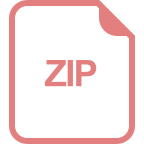
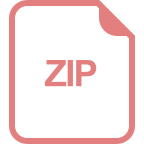
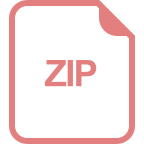
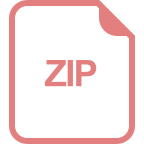
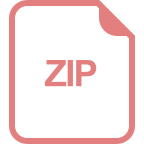