用文件存放迷宫地图,实现迷宫游戏代码C语言
时间: 2023-07-16 16:15:01 浏览: 174
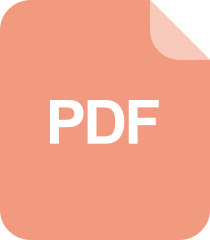
基于C语言实现的迷宫游戏代码
以下是一个简单的迷宫游戏代码示例,使用文件存放迷宫地图。假设地图文件为maze.txt,格式为:
```
10 10
##########
# #
# ###### #
# # #
# # #### #
# # # #
# # # ####
# # #
### # ####
# e #
```
其中,第一行为地图的行数和列数,后面的字符表示地图中的墙壁(#)、空地(空格)和终点(e)。
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_ROWS 100
#define MAX_COLS 100
// 地图结构体
typedef struct {
int rows; // 行数
int cols; // 列数
char map[MAX_ROWS][MAX_COLS]; // 地图数组
} Maze;
// 读取地图
void read_map(const char *filename, Maze *maze) {
FILE *fp;
int i, j;
// 打开文件
fp = fopen(filename, "r");
if (fp == NULL) {
fprintf(stderr, "Error: cannot open file %s.\n", filename);
exit(EXIT_FAILURE);
}
// 读取地图大小
fscanf(fp, "%d %d", &maze->rows, &maze->cols);
if (maze->rows <= 0 || maze->rows > MAX_ROWS || maze->cols <= 0 || maze->cols > MAX_COLS) {
fprintf(stderr, "Error: invalid map size.\n");
exit(EXIT_FAILURE);
}
// 读取地图数据
for (i = 0; i < maze->rows; i++) {
for (j = 0; j < maze->cols; j++) {
maze->map[i][j] = fgetc(fp);
if (maze->map[i][j] == EOF) {
fprintf(stderr, "Error: invalid map data.\n");
exit(EXIT_FAILURE);
}
if (maze->map[i][j] == '\n') {
j--;
}
}
}
// 关闭文件
fclose(fp);
}
// 打印地图
void print_map(const Maze *maze) {
int i, j;
for (i = 0; i < maze->rows; i++) {
for (j = 0; j < maze->cols; j++) {
printf("%c", maze->map[i][j]);
}
printf("\n");
}
}
// 游戏主循环
void game_loop(Maze *maze) {
int x = 1, y = 1; // 初始位置
int dx, dy; // 移动距离
char input; // 输入字符
// 清屏
system("clear");
// 打印地图
print_map(maze);
// 循环读取用户输入并移动
while (1) {
// 读取输入
input = getchar();
// 处理输入
switch (input) {
case 'w':
dx = -1;
dy = 0;
break;
case 'a':
dx = 0;
dy = -1;
break;
case 's':
dx = 1;
dy = 0;
break;
case 'd':
dx = 0;
dy = 1;
break;
case 'q':
return; // 退出游戏
default:
dx = 0;
dy = 0;
break;
}
// 移动位置
if (maze->map[x+dx][y+dy] != '#') {
maze->map[x][y] = ' ';
x += dx;
y += dy;
maze->map[x][y] = 'o';
}
// 清屏
system("clear");
// 打印地图
print_map(maze);
// 判断是否到达终点
if (maze->map[x][y] == 'e') {
printf("Congratulations! You win!\n");
return;
}
}
}
int main() {
Maze maze;
// 读取地图
read_map("maze.txt", &maze);
// 进入游戏循环
game_loop(&maze);
return 0;
}
```
阅读全文
相关推荐
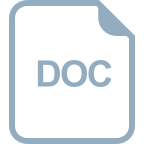
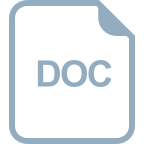
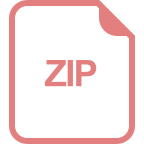
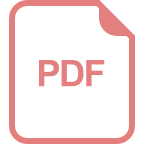
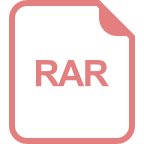
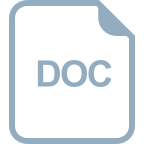
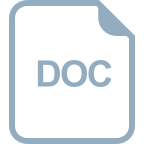
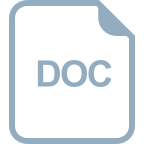
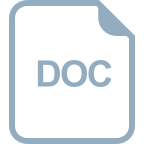
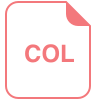
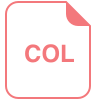