请实现基于Android studio 开发的用户登陆界面
时间: 2023-11-22 10:56:13 浏览: 161
好的,以下是基于Android Studio开发的用户登录界面的示例代码:
1. 在XML布局文件中添加以下代码(文件名为activity_login.xml):
```xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="200dp"
android:layout_height="200dp"
android:layout_marginTop="50dp"
android:background="@drawable/ic_launcher_foreground"
android:layout_centerHorizontal="true"/>
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/imageView"
android:layout_centerHorizontal="true"
android:layout_marginTop="30dp"
android:text="User Login"
android:textSize="24sp"/>
<EditText
android:id="@+id/editTextUsername"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_below="@+id/textView"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp"
android:hint="Username"/>
<EditText
android:id="@+id/editTextPassword"
android:layout_width="300dp"
android:layout_height="wrap_content"
android:layout_below="@+id/editTextUsername"
android:layout_centerHorizontal="true"
android:layout_marginTop="20dp"
android:hint="Password"
android:inputType="textPassword"/>
<Button
android:id="@+id/buttonLogin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/editTextPassword"
android:layout_centerHorizontal="true"
android:layout_marginTop="40dp"
android:background="@color/colorPrimary"
android:text="Login"
android:textColor="@android:color/white"/>
</RelativeLayout>
```
2. 在Java文件中添加以下代码(文件名为LoginActivity.java):
```java
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
public class LoginActivity extends AppCompatActivity {
EditText editTextUsername, editTextPassword;
Button buttonLogin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
editTextUsername = findViewById(R.id.editTextUsername);
editTextPassword = findViewById(R.id.editTextPassword);
buttonLogin = findViewById(R.id.buttonLogin);
buttonLogin.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
String username = editTextUsername.getText().toString().trim();
String password = editTextPassword.getText().toString().trim();
if (username.isEmpty()) {
Toast.makeText(getApplicationContext(), "Please enter username", Toast.LENGTH_SHORT).show();
} else if (password.isEmpty()) {
Toast.makeText(getApplicationContext(), "Please enter password", Toast.LENGTH_SHORT).show();
} else if (!username.equals("admin") || !password.equals("admin")) {
Toast.makeText(getApplicationContext(), "Invalid username or password", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(getApplicationContext(), "Login successful", Toast.LENGTH_SHORT).show();
// enter your code here for successful login
}
}
});
}
}
```
以上代码中,我们首先在onCreate()方法中获取了EditText和Button的实例。当用户点击登录按钮时,我们检查了用户名和密码是否为空,并且验证了它们是否与预定义的值匹配。如果验证成功,我们可以在成功登录的代码块中添加自己的代码。
3. 最后,将LoginActivity设置为我们的启动活动(即MainActivity),并运行应用程序即可。
注意:此示例代码仅用于演示目的,并不是一个完整的、安全的登录系统。在实际应用中,您需要更加复杂的验证和安全措施来保护用户数据。
阅读全文
相关推荐
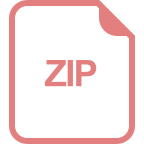
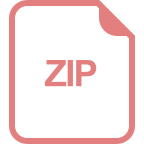
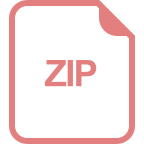
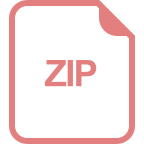
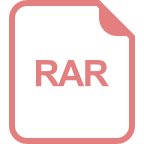
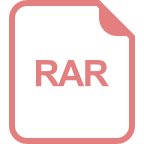
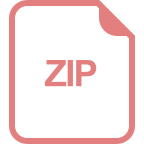
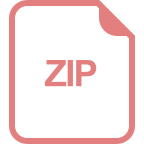
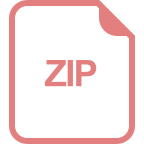
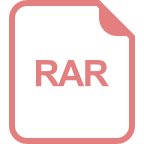
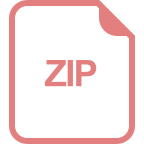
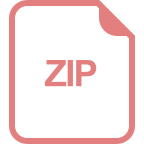
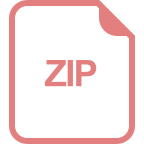
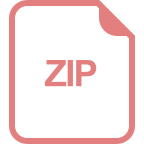
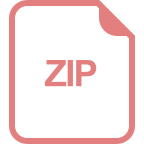
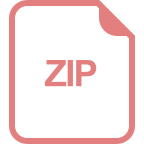