springboot 邮箱发送群发并且发送多个附件
时间: 2023-06-28 10:08:35 浏览: 312
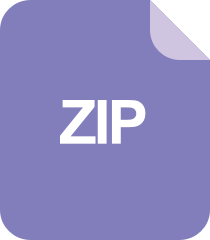
SpringBoot中整合Mail实现发送带附件的邮件示例代码
在 Spring Boot 中,实现邮箱的群发和发送多个附件可以使用 JavaMail API。下面是一个简单的示例代码:
```java
import java.io.File;
import java.util.Properties;
import javax.mail.Authenticator;
import javax.mail.Message;
import javax.mail.MessagingException;
import javax.mail.Session;
import javax.mail.Transport;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeBodyPart;
import javax.mail.internet.MimeMessage;
import javax.mail.internet.MimeMultipart;
import javax.mail.internet.MimeUtility;
public class EmailService {
private String host; // SMTP服务器主机名
private String username; // 发件人账号
private String password; // 发件人密码
public EmailService(String host, String username, String password) {
this.host = host;
this.username = username;
this.password = password;
}
public void sendEmail(String[] to, String[] cc, String[] bcc, String subject, String content, String[] attachments) throws Exception {
Properties props = new Properties();
props.put("mail.smtp.host", host);
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.ssl.enable", "true");
Authenticator auth = new EmailAuthenticator(username, password);
Session mailSession = Session.getInstance(props, auth);
MimeMessage message = new MimeMessage(mailSession);
message.setFrom(new InternetAddress(username));
InternetAddress[] toAddresses = new InternetAddress[to.length];
for (int i = 0; i < to.length; i++) {
toAddresses[i] = new InternetAddress(to[i]);
}
message.setRecipients(Message.RecipientType.TO, toAddresses);
if (cc != null && cc.length > 0) {
InternetAddress[] ccAddresses = new InternetAddress[cc.length];
for (int i = 0; i < cc.length; i++) {
ccAddresses[i] = new InternetAddress(cc[i]);
}
message.setRecipients(Message.RecipientType.CC, ccAddresses);
}
if (bcc != null && bcc.length > 0) {
InternetAddress[] bccAddresses = new InternetAddress[bcc.length];
for (int i = 0; i < bcc.length; i++) {
bccAddresses[i] = new InternetAddress(bcc[i]);
}
message.setRecipients(Message.RecipientType.BCC, bccAddresses);
}
message.setSubject(subject);
MimeMultipart contentPart = new MimeMultipart();
MimeBodyPart textPart = new MimeBodyPart();
textPart.setText(content);
contentPart.addBodyPart(textPart);
if (attachments != null && attachments.length > 0) {
for (String attachment : attachments) {
MimeBodyPart attachPart = new MimeBodyPart();
File file = new File(attachment);
attachPart.attachFile(file);
attachPart.setFileName(MimeUtility.encodeText(file.getName()));
contentPart.addBodyPart(attachPart);
}
}
message.setContent(contentPart);
Transport.send(message);
}
private static class EmailAuthenticator extends Authenticator {
private String username;
private String password;
public EmailAuthenticator(String username, String password) {
this.username = username;
this.password = password;
}
@Override
protected javax.mail.PasswordAuthentication getPasswordAuthentication() {
return new javax.mail.PasswordAuthentication(username, password);
}
}
}
```
然后就可以在你的代码中使用这个 EmailService 类来发送邮件了:
```java
EmailService emailService = new EmailService("smtp.example.com", "username@example.com", "password");
String[] to = {"recipient1@example.com", "recipient2@example.com"};
String[] cc = {"cc1@example.com", "cc2@example.com"};
String[] bcc = {"bcc1@example.com", "bcc2@example.com"};
String subject = "Test email";
String content = "This is a test email.";
String[] attachments = {"/path/to/attachment1", "/path/to/attachment2"};
emailService.sendEmail(to, cc, bcc, subject, content, attachments);
```
其中,`to`、`cc`、`bcc` 分别表示邮件的收件人、抄送人、密送人,`subject` 表示邮件主题,`content` 表示邮件内容,`attachments` 表示邮件的附件路径数组。
阅读全文
相关推荐
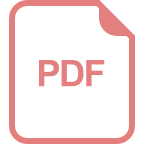
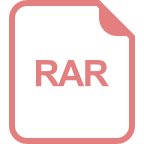
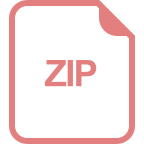
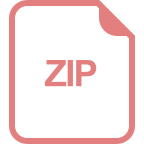
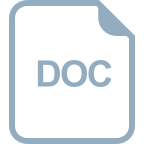



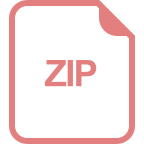
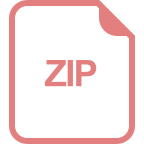
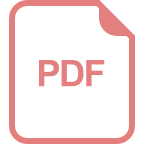
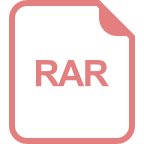
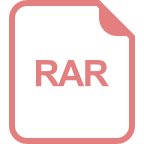